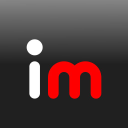
ImgFlip
PhotographyAPI Documentation for Imgflip
Imgflip is a website that offers a range of meme creation tools and features. You can access Imgflip's API to programmatically generate meme content using code. In this blog post, we'll explore how to use the Imgflip API in JavaScript with code examples.
Getting Started
To use the Imgflip API, you need to sign up for an account and get an API key. The API key is required for every API request you make, so make sure to keep it safe. After you have your API key, you can start making API requests.
Example Code
const imgflipUsername = '<YOUR_IMGFLIP_USERNAME>';
const imgflipPassword = '<YOUR_IMGFLIP_PASSWORD>';
const imgflipAPIKey = '<YOUR_IMGFLIP_API_KEY>';
// Example API Request using Axios
const axios = require('axios');
async function getMemes() {
const res = await axios.get(
`https://api.imgflip.com/get_memes`,
);
// Do something with the response data
console.log(res.data)
}
// Example API Request using fetch
function generateMeme(templateID, text0, text1) {
const url = `https://api.imgflip.com/caption_image?template_id=${templateID}&text0=${text0}&text1=${text1}&username=${imgflipUsername}&password=${imgflipPassword}&api_key=${imgflipAPIKey}`;
fetch(url)
.then(res => res.json())
.then(data => {
// Do something with the response data
console.log(data);
})
.catch(err => console.log(err));
}
// Example API Request using jQuery
function searchMemes(query) {
const url = `https://api.imgflip.com/search?q=${query}&username=${imgflipUsername}&password=${imgflipPassword}&api_key=${imgflipAPIKey}`;
$.get(url)
.done(res => {
// Do something with the response data
console.log(res);
})
.fail(err => console.log(err));
}
API Endpoints
The Imgflip API has various endpoints that you can use to perform different actions, such as getting a list of meme templates, generating a meme image, or searching for memes.
Example Code
// Get a list of meme templates
async function getMemeTemplates() {
const res = await axios.get(
`https://api.imgflip.com/get_memes`,
);
// Do something with the response data
console.log(res.data)
}
// Generate a meme image
function generateMeme(templateID, text0, text1) {
const url = `https://api.imgflip.com/caption_image?template_id=${templateID}&text0=${text0}&text1=${text1}&username=${imgflipUsername}&password=${imgflipPassword}&api_key=${imgflipAPIKey}`;
fetch(url)
.then(res => res.json())
.then(data => {
// Do something with the response data
console.log(data);
})
.catch(err => console.log(err));
}
// Search for memes
function searchMemes(query) {
const url = `https://api.imgflip.com/search?q=${query}&username=${imgflipUsername}&password=${imgflipPassword}&api_key=${imgflipAPIKey}`;
$.get(url)
.done(res => {
// Do something with the response data
console.log(res);
})
.fail(err => console.log(err));
}
Conclusion
In this blog post, we explored the Imgflip API and how to use it with JavaScript code examples. With this information, you can start creating your own memes programmatically using Imgflip's API. We hope this was helpful!