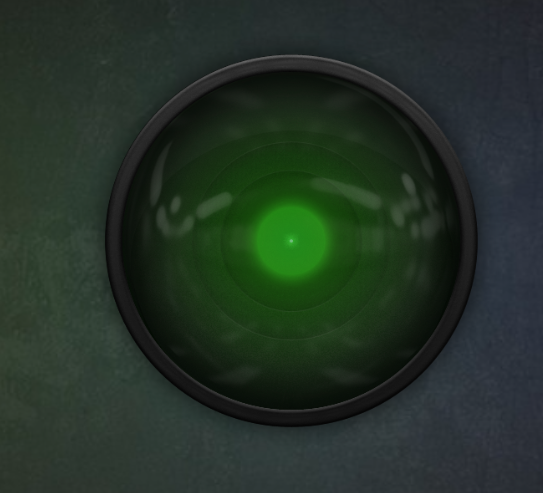
inspirobot
Art & DesignHow to Use Inspirobot API in JavaScript
If you are looking for a motivational quote or an inspirational image, then Inspirobot API is a great option. It is a free-to-use API that provides random motivational quotes and images to inspire and motivate people. In this article, we will see how to use Inspirobot API in JavaScript.
Step 1: Get the API Key
To use Inspirobot API, you need an API key. You can get it by visiting their website, where you can find the API documentation and a button to generate the API key. Once you have your API key, you can start using the API.
Step 2: Make an API Request
You can make a GET request to the Inspirobot API to get a random motivational quote or an image. Here is an example code to get a motivational quote:
const apiKey = 'your_api_key';
const apiEndpoint = 'https://inspirobot.me/api';
fetch(`${apiEndpoint}/?generate=true&api_key=${apiKey}`)
.then(response => response.text())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In the above code, we are using fetch
to make an API request. We are also passing the generate
and api_key
parameters to the API endpoint. The generate
parameter is set to true
to get a random quote. The api_key
parameter is your unique API key.
After making the API request, you will get a response with a link to a motivational quote image. You can display this image on your webpage using the following code:
const img = document.createElement('img');
img.src = data.url;
document.body.appendChild(img);
In the above code, we are creating an img
element and setting the src
attribute to the URL of the motivational quote image.
Step 3: Handle Errors
It is always a good practice to handle errors when making API requests. Here is an example code to handle errors:
fetch(`${apiEndpoint}/?generate=true&api_key=${apiKey}`)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.text();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In the above code, we are checking the ok
property of the response object to see if the API request was successful. If it is not successful, we are throwing an error.
Conclusion
Using Inspirobot API is very easy and a great way to add some motivation and inspiration to your website. In this article, we have seen how to make an API request and display the motivational quote image on your webpage. We have also seen how to handle errors when making API requests. So, go ahead and add some inspiration to your website using the Inspirobot API.