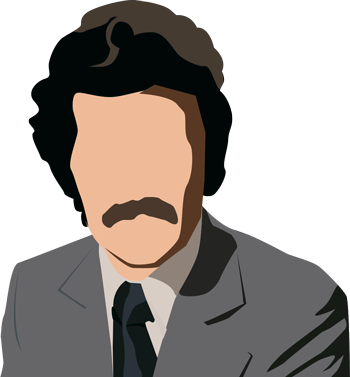
jService - Trivia API
Games & ComicsIntroduction to JService API
JService.io is a simple and free API that provides a database of over 200,000 Jeopardy questions and answers. You can use JService.io to create your own Jeopardy game, trivia app or other Jeopardy-themed projects. In this blog post, we will explore the different API endpoints provided by JService.io and how to use them in your JavaScript application.
Getting Started
Before we can start using JService.io, we need to get an API token. You can obtain the token by visiting the JService.io website and clicking on the "Get API Token" button. Once you have your API token, you can start making API requests.
API Endpoints
JService.io provides several API endpoints that you can use to retrieve Jeopardy questions. Let's explore these endpoints and how to use them in your JavaScript application.
Random Question Endpoint
The first endpoint we will explore is the random question endpoint. This endpoint allows you to retrieve a random Jeopardy question. Here is an example code snippet that demonstrates how to use the random question endpoint:
fetch('http://jservice.io/api/random')
.then(response => response.json())
.then(data => console.log(data[0]))
.catch(error => console.error(error));
In this code snippet, we use the fetch()
function to make a GET request to the random question endpoint. The response returned by the endpoint is in JSON format, so we use the json()
method to parse the response. The data
object returned by the API contains an array of questions. In this case, we only want to display the first question in the array.
Category Endpoint
The category endpoint allows you to retrieve questions from a specific Jeopardy category. Here is an example code snippet that demonstrates how to use the category endpoint:
fetch('http://jservice.io/api/category?id=1')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this code snippet, we use the fetch()
function to make a GET request to the category endpoint. The id
parameter specifies the ID of the category we want to retrieve questions from. The response returned by the endpoint is in JSON format, so we use the json()
method to parse the response. The data
object returned by the API contains an array of questions from the specified category.
Search Endpoint
The search endpoint allows you to retrieve Jeopardy questions that match a specific search term. Here is an example code snippet that demonstrates how to use the search endpoint:
fetch('http://jservice.io/api/search?query=computer')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this code snippet, we use the fetch()
function to make a GET request to the search endpoint. The query
parameter specifies the search term we want to search for. The response returned by the endpoint is in JSON format, so we use the json()
method to parse the response. The data
object returned by the API contains an array of questions that match the search term.
Conclusion
In this blog post, we explored the different API endpoints provided by JService.io and how to use them in your JavaScript application. JService.io provides a simple and free way to access a large database of Jeopardy questions and answers. If you are building a Jeopardy-themed project, JService.io is definitely an API worth considering.