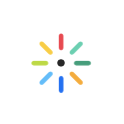
Kaltura API
VideoLearn Video API with Kaltura's Public API Docs
Kaltura's Video Platform as a Service (PaaS) offers an extensive public API that can be accessed using various programming languages. In this blog post, we'll focus on using JavaScript to access Kaltura's Video PaaS API.
Getting Started
Before we dive into specific examples, you'll need to obtain an API key from Kaltura. You can do this by following these instructions.
Once you've obtained your API key, you'll be able to use the API endpoint https://www.kaltura.com/api_v3/
to access the various API actions.
Uploading a Video
One of the most essential features of Kaltura's Video PaaS is uploading videos. To upload a video using JavaScript, you'll need to use the XMLHttpRequest
object to make a POST
request to the designated API action.
const xhr = new XMLHttpRequest();
xhr.open("POST", "https://www.kaltura.com/api_v3/?service=uploadtoken&action=add", true);
const formData = new FormData();
formData.append("format", "1");
formData.append("ks", "INSERT_YOUR_API_KEY_HERE");
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) {
console.log(xhr.responseText);
}
};
xhr.send(formData);
Retrieving Data
You can also use JavaScript to retrieve data from Kaltura's Video PaaS. For example, if you want to retrieve a list of all categories on your account, you can make a GET
request to the API endpoint with the service
parameter set to category
and the action
parameter set to list
.
const xhr = new XMLHttpRequest();
xhr.open("GET", "https://www.kaltura.com/api_v3/?service=category&action=list&ks=INSERT_YOUR_API_KEY_HERE");
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) {
console.log(xhr.responseText);
}
};
xhr.send();
Updating Data
JavaScript can also be used to update data on Kaltura's Video PaaS platform. For example, if you want to update the name of a specific video, you can make a POST
request to the media
API action update
with the ID of the video and the new name.
const xhr = new XMLHttpRequest();
xhr.open("POST", "https://www.kaltura.com/api_v3/?service=media&action=update&entryId=INSERT_VIDEO_ID_HERE&name=NEW_VIDEO_NAME&ks=INSERT_YOUR_API_KEY_HERE", true);
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) {
console.log(xhr.responseText);
}
};
xhr.send();
Conclusion
This blog post has demonstrated how to use JavaScript to access Kaltura's Video PaaS API. We've covered uploading videos, retrieving data, and updating data. By utilizing these examples, you can extend Kaltura's Video PaaS functionality to your web applications.