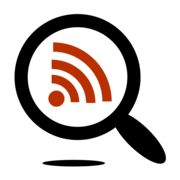
Listen Notes API
Open DataGetting Started with Listen Notes API
Listen Notes API is a comprehensive platform that allows you to search, retrieve, and manipulate podcasts, episodes, and other podcast-related information. In this blog post, we will take a look at the various features offered by Listen Notes API and how you can use JavaScript to interact with the API.
Prerequisites
Before we dive into the coding part, we need to make sure that we have an API key. To obtain an API key, you need to sign up at Listen Notes API website.
API Endpoints
Listen Notes API provides various endpoints to interact with podcast-related information. The following is the list of endpoints supported by Listen Notes API:
- Search API
- Typeahead API
- Fetching Metadata of a Podcast
- Fetching Metadata of an Episode
- Apple Podcasts Lookup API
We will include code snippets to demonstrate the usage of the above endpoints.
Search API
The Search API allows you to search for podcasts with specific keywords. The following code snippet demonstrates how to use the Search API in JavaScript:
const fetch = require('node-fetch');
const searchPodcasts = async () => {
const url = 'https://listen-api.listennotes.com/api/v2/search?q=javascript';
const response = await fetch(url, {
headers: {
'X-ListenAPI-Key': 'Your-API-Key-Here'
}
});
const data = await response.json();
console.log(data);
}
searchPodcasts();
You can replace "Your-API-Key-Here" with your Listen Notes API key.
Typeahead API
The Typeahead API is similar to the Search API but returns suggestions as you type, rather than returning a list of matching results. The following code snippet demonstrates how to use the Typeahead API in JavaScript:
const fetch = require('node-fetch');
const getTypeahead = async() => {
const url = 'https://listen-api.listennotes.com/api/v2/typeahead?q=famous&type=podcast'
const response = await fetch(url, {
headers: {
'X-ListenAPI-Key': 'Your-API-Key-Here'
}
});
const data = await response.json();
console.log(data);
}
getTypeahead();
Fetching Metadata of a Podcast
The "podcast" endpoint allows you to retrieve metadata for a specific podcast. The following code snippet demonstrates how to use the "podcast" endpoint in JavaScript:
const fetch = require('node-fetch');
const getPodcast = async () => {
const url = 'https://listen-api.listennotes.com/api/v2/podcasts/5a669767b8a7726f6fa62d2e5b26b37e/';
const response = await fetch(url, {
headers: {
'X-ListenAPI-Key': 'Your-API-Key-Here'
}
});
const data = await response.json();
console.log(data);
}
getPodcast();
Fetching Metadata of an Episode
The "episodes" endpoint allows you to retrieve metadata for a specific podcast episode. The following code snippet demonstrates how to use the "episodes" endpoint in JavaScript:
const fetch = require('node-fetch');
const getEpisode = async () => {
const url = 'https://listen-api.listennotes.com/api/v2/episodes/90fae2e194784d9f8ace5f46c236df2c/';
const response = await fetch(url, {
headers: {
'X-ListenAPI-Key': 'Your-API-Key-Here'
}
});
const data = await response.json();
console.log(data);
}
getEpisode();
Apple Podcasts Lookup API
The "apple_podcasts_lookup" endpoint allows you to retrieve Apple Podcasts metadata for a specific Apple Podcasts ID. The following code snippet demonstrates how to use the "apple_podcasts_lookup" endpoint in JavaScript:
const fetch = require('node-fetch');
const getApplePodcasts = async () => {
const url = 'https://listen-api.listennotes.com/api/v2/apple_podcasts_lookup?id=975332830';
const response = await fetch(url, {
headers: {
'X-ListenAPI-Key': 'Your-API-Key-Here'
}
});
const data = await response.json();
console.log(data);
}
getApplePodcasts();
Conclusion
Listen Notes API is a powerful platform that allows you to interact with podcasts, episodes, and other related information. In this blog post, we took a look at the various API endpoints supported by Listen Notes API and how to use JavaScript to interact with the endpoints. Remember to replace "Your-API-Key-Here" with your Listen Notes API key.