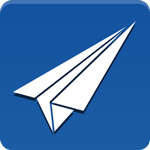
mailboxlayer API
Data ValidationUsing MailboxLayer API in JavaScript
MailboxLayer is a powerful REST API that provides email validation and verification services for developers. In this blog post, we will explore the mailboxlayer API and show you how to use it to validate email addresses using JavaScript.
Getting started
To get started, you will need an API key. You can sign up for a free API key from the mailboxlayer website. Once you have your API key, you can start using the mailboxlayer API.
API Endpoint
The API endpoint for mailboxlayer is https://apilayer.net/api/check
. You will need to append your API key to the endpoint as a query parameter like this: https://apilayer.net/api/check?access_key=YOUR_ACCESS_KEY&email=EMAIL_TO_VERIFY
.
Sending a request
To send a request to the mailboxlayer API, you can use JavaScript's built-in fetch
function. Here's an example code snippet to send a request and retrieve a response:
const email = 'support@mailboxlayer.com';
const apiKey = 'YOUR_API_KEY';
const endpoint = `https://apilayer.net/api/check?access_key=${apiKey}&email=${email}`;
fetch(endpoint)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
This code snippet sends a request to the mailboxlayer API with a sample email address 'support@mailboxlayer.com'. We append our API key to the endpoint and add the email query parameter to verify it. On success, we log the response in the console, and on failure, we log the error.
Parsing response
The mailboxlayer API response includes various information and statuses about the email address you want to verify. Here's an example of how you can parse the response:
.then(data => {
const {
format_valid,
smtp_check,
score,
country_code,
domain,
mx_found
} = data;
console.log('Format Valid:', format_valid);
console.log('SMTP Check:', smtp_check);
console.log('Score:', score);
console.log('Country Code:', country_code);
console.log('Domain:', domain);
console.log('MX Found:', mx_found);
})
In this code snippet, we destructure some key values from the mailboxlayer API response object. We then log them in the console, so we can see the email address's validation status.
Conclusion
In conclusion, mailboxlayer is a powerful email validation API that provides an easy to use REST API for developers. Using JavaScript, we can quickly integrate mailboxlayer into our web applications, and validate email addresses to ensure they are valid and trustworthy.