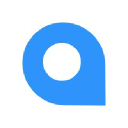
đ Documentation & Examples
Everything you need to integrate with US Autocomplete
đ Quick Start Examples
// US Autocomplete API Example
const response = await fetch('https://www.smarty.com/docs/cloud/us-autocomplete-pro-api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
The Smarty US Autocomplete Pro API is a robust tool designed to streamline and optimize the process of inputting address data. By providing real-time suggestions, it reduces the potential for error and significantly boosts the speed of operation. As user types in an address, suggestive autofill kicks in, refining the options based on the entered characters. This feature can be particularly beneficial for businesses with vast customer databases where address accuracy is essential. Detailed documentation on how to fully utilize this powerful API can be found here.
There are numerous advantages to using the US Autocomplete Pro API, including:
- Increased speed: By suggesting addresses in real-time, the API minimizes the time spent on inputting data.
- Enhanced accuracy: The intuitive autofill reduces the likelihood of any address errors.
- Better user experience: It improves your app's UX by making data entry faster and more efficient.
- Reduced cart abandonment rate: By speeding up the checkout process, it directly impacts your bottom line.
- Versatility: The API can be used for various applications, like e-commerce, delivery services, or any business that rely on accurate address data.
Here's a simple JavaScript code example on how to call the Smarty US Autocomplete Pro API:
const axios = require('axios');
const instance = axios.create({
baseURL: 'https://api.smarty.com/',
timeout: 1000,
headers: {'Authentication-Key': 'YOUR_AUTH_KEY'}
});
instance.get('/us-autocomplete-pro-api?input={address}')
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.log(error);
});
In the code above, remember to replace 'YOUR_AUTH_KEY'
with your actual authentication key, and {address}
should be replaced with the address string that you want to autocomplete.
Security Assessment
đ 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes