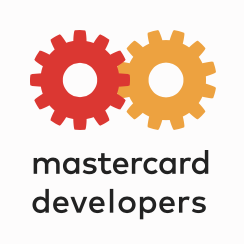
mastercard
FinanceMastercard Developer API Docs
Mastercard Developer is a platform that provides access to a variety of APIs, allowing developers to build new applications and services that use Mastercard data and services. This platform makes it easy to integrate Mastercard's capabilities into your applications and create innovative solutions with ease.
To get started with the Mastercard Developer API Docs, you'll need to create an account and explore the available APIs. Once you have access to an API, you can start making requests and receiving responses that contain the data or functionality you need for your application.
Example JavaScript Code
Here are some example code snippets in JavaScript that show how to make requests to the Mastercard APIs. These examples assume that you have already created an account and obtained an API key for the APIs you want to use.
Getting Started
Before you can start making requests to the Mastercard APIs, you'll need to include the relevant JavaScript libraries in your application. Here is an example:
<script src="https://apigateway.mastercard.com/mastercard-api-client.js"></script>
This library provides an easy-to-use interface for making requests to the Mastercard APIs. You can then use the following code to initialize the API client with your API key:
var api = new MastercardAPI.Client({
apiKey: "YOUR API KEY HERE",
sandbox: true // Use this for testing purposes only
});
Location API Example
The Mastercard Location API allows you to retrieve information about businesses and points of interest based on location data. Here is some example code that shows how to use this API:
api.location("", {
latitude: "40.7128",
longitude: "74.0059",
country: "US",
radius: "2",
pageOffset: "0",
pageLength: "10"
}, function(response) {
console.log(response); // Do something with the response data
});
This code makes a request to the Mastercard Location API to retrieve businesses and points of interest that are located within 2 miles of the specified latitude and longitude coordinates.
Fraud Detection API Example
The Mastercard Fraud Detection API allows you to detect and prevent fraud by analyzing payment transactions in real-time. Here is some example code that shows how to use this API:
api.fraud.detect({
"request": {
"audience": "internal",
"eventType": "paymentTransaction",
"event": {
"accountNumber": "1234567890123456",
"amount": "100.00",
"currency": "USD"
}
}
}, function(response) {
console.log(response); // Do something with the response data
});
This code makes a request to the Mastercard Fraud Detection API to analyze a payment transaction and determine if it is fraudulent.
Conclusion
The Mastercard Developer API Docs provide an easy way for developers to integrate Mastercard's capabilities into their applications. With the help of the example code snippets provided in this blog, you can quickly get started with the APIs and start building innovative new solutions that leverage Mastercard data and services.