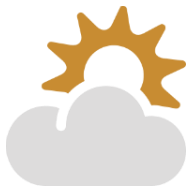
MetaWeather
WeatherAccessing Weather Data with the MetaWeather API
Are you tired of checking weather updates every day? Do you want an easy way to access accurate weather data for multiple locations? Look no further than the MetaWeather API!
This public API provides weather data for a variety of locations and timeframes, making it a valuable tool for developers who need to incorporate weather data into their applications.
How to Use the MetaWeather API
First, you will need to obtain an API key from the MetaWeather website. Once you have that, you can start making requests to the API.
There are several endpoints available, including:
- Location Search: find locations based on a search term
- Location Information: get detailed information about a specific location
- Location Weather: get weather data for a specific location
To use any of these endpoints, you will need to construct a URL with the appropriate parameters and make a GET request to the API.
Here are some example code snippets in JavaScript:
Location Search Example
const endpoint = 'https://www.metaweather.com/api/location/search/';
const query = 'new york';
fetch(`${endpoint}?query=${query}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code makes a request to the Location Search endpoint, searching for locations that match the query "new york". The response contains an array of objects, each representing a location that matches the query.
Location Information Example
const endpoint = 'https://www.metaweather.com/api/location/';
const locationId = '2459115';
fetch(`${endpoint}${locationId}/`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code makes a request to the Location Information endpoint, requesting information about the location with ID 2459115 ("New York City"). The response contains detailed information about the location, including its name, timezone, and geographic coordinates.
Location Weather Example
const endpoint = 'https://www.metaweather.com/api/location/';
const locationId = '2459115';
fetch(`${endpoint}${locationId}/`)
.then(response => response.json())
.then(data => {
const consolidatedWeather = data.consolidated_weather[0];
console.log(`The weather in ${data.title} is currently ${consolidatedWeather.weather_state_name.toLowerCase()} with a temperature of ${consolidatedWeather.the_temp}°C.`);
})
.catch(error => console.error(error));
This code makes a request to the Location Weather endpoint, requesting current weather information for the location with ID 2459115 ("New York City"). The response contains an array of weather data objects, with the most recent data at index 0. In this example, we log the current weather conditions for the location.
Conclusion
The MetaWeather API provides a useful tool for accessing weather data for a variety of locations and timeframes. By constructing URLs with appropriate parameters and making GET requests using JavaScript, you can easily incorporate weather data into your applications. Happy coding!