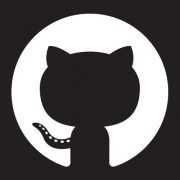
Mexico
Open DataUsing the SEPOMEX API with JavaScript
The SEPOMEX API is a public API that provides access to the Mexican Postal Service’s database of postal codes. It is a great resource for developers building applications that might require the use of postal codes. This tutorial will guide you through the process of using the SEPOMEX API with JavaScript, and provide you with some example code to help you get started.
Getting Started
To use the SEPOMEX API, you’ll need to first create an account, and then obtain an API key. Once you have your API key, you can start sending requests to the SEPOMEX API.
Sending Requests
To send requests to the SEPOMEX API, you can use the fetch
function that is built into JavaScript. Here is an example of how to use fetch
to send a request to the API to obtain a list of all the postal codes for a given state:
fetch('https://sepomex.icalialabs.com/api/v1/states/NAY/postal-codes', {
headers: {
'api-key': 'YOUR_API_KEY_HERE'
}
}).then((response) => {
return response.json();
}).then((data) => {
console.log(data);
}).catch((error) => {
console.error(error);
});
In this example, we are sending a GET request to the URL https://sepomex.icalialabs.com/api/v1/states/NAY/postal-codes
, which will return a list of all the postal codes for the state of Nayarit. We are also including our API key in the headers of the request.
When we receive a response from the API, we are parsing the data into a JSON format, and then logging it to the console. If there is an error, we are logging the error to the console as well.
Conclusion
The SEPOMEX API is a useful resource for developers building applications that need to use postal codes in Mexico. With just a few lines of JavaScript, you can send requests to the API and retrieve the data you need. We hope this tutorial has helped you get started with using the SEPOMEX API with JavaScript.