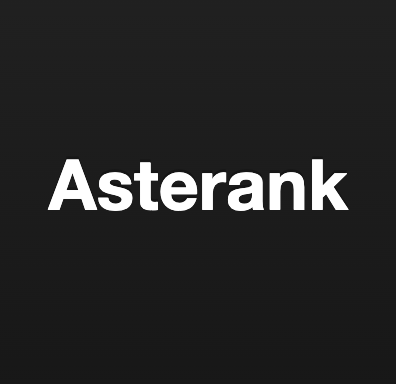
Minor Planet Center
Open DataExploring the Solar System with Asterank API
Have you ever wondered about the composition and properties of objects in our Solar System? Well, now you can access that information through the Asterank API. Asterank is a free API that provides data on asteroids, comets, and dwarf planets in our Solar System. In this blog post, we will explore how to use this API, with examples in JavaScript.
Getting Started
First, you need to obtain an API key by creating an account on their website. This will allow you to access the full power of the Asterank API. Once you have your key, you can start making requests to the API.
API Endpoints
The Asterank API has several endpoints that you can use to retrieve data about Solar System objects. Some of the most commonly used ones are:
browse
: retrieve a list of objects matching specific criteria, such as closest approach to Earth or mass.search
: search for an object by name or id.object
: retrieve detailed information about a specific object.mpc
: retrieve basic information about objects from the Minor Planet Center database.
In this post, we will focus on the mpc
endpoint, which is the easiest one to use.
Using the MPC Endpoint
The mpc
endpoint allows you to retrieve basic information about objects in the Minor Planet Center database. You can retrieve information about a single object or a group of objects that match certain criteria.
Let's start with a basic example of retrieving information about a single object:
const apiKey = 'YOUR_API_KEY';
const objectId = '2000433';
fetch(`http://www.asterank.com/mpc?query={"id":"${objectId}"}&api_key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we are retrieving information about the asteroid with the id 2000433
. We are using the fetch
function to make a GET request to the mpc
endpoint, with a query parameter that specifies the object id we are interested in. We also pass our API key as a query parameter. Finally, we parse the response as JSON and log it to the console.
The response we get back will look like this:
[{
"i": 10.8207,
"e": 0.207695,
"a": 2.76948,
"q": 2.19292,
"period_days": 4.6,
"id": "2000433",
"full_name": "2000433 1990 RP5",
"prov_des": "1990 RP5",
"name": null,
"orbit_id": null,
"pdes": null
}]
This response contains the basic orbital parameters of the object, as well as some identification information.
Next, let's see how to retrieve a group of objects that match certain criteria:
const apiKey = 'YOUR_API_KEY';
const minDiameter = 10;
const maxDistance = 1;
fetch(`http://www.asterank.com/mpc?query={"diameter":{"$gt":${minDiameter}},"distance":{"$lt":${maxDistance}}}&limit=10&api_key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we are retrieving a list of up to 10 objects that have a diameter greater than 10 kilometers and are closer than 1 astronomical unit (AU) to Earth. We are using the $gt
and $lt
operators to filter the objects based on their properties. We also specify the limit
parameter to limit the number of objects we retrieve. The rest of the code is similar to the previous example.
The response we get back will look something like this:
[{
"i": 4.49237,
"e": 0.430162,
"a": 4.0678,
"q": 2.3221,
"period_days": 5.149,
"id": "385343",
"full_name": "385343 2001 RX14",
"prov_des": "2001 RX14",
"name": null,
"orbit_id": null,
"pdes": null
},
{
"i": 7.2036,
"e": 0.0657997,
"a": 1.36884,
"q": 1.27761,
"period_days": 1.61,
"id": "2002 JF56",
"full_name": "2002 JF56",
"prov_des": "2002 JF56",
"name": null,
"orbit_id": null,
"pdes": null
},
...
]
This response contains a list of objects that match the criteria we specified, each with its own set of orbital parameters and identification information.
Conclusion
In this post, we explored how to use the Asterank API to retrieve basic information about objects in our Solar System. We focused on the mpc
endpoint, which allows us to retrieve information from the Minor Planet Center database. We saw how to retrieve information about a single object and a group of objects that match specific criteria. With this API, you can start exploring the Solar System and discovering new things about the objects that inhabit it.