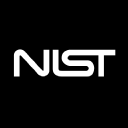
National Vulnerability Database
SecurityWorking with NIST National Vulnerability Database JSON Feed
The National Vulnerability Database (NVD) is a repository of standards-based vulnerability management data represented using the Security Content Automation Protocol (SCAP). SCAP incorporates a suite of specifications including descriptions of vulnerability names, severity, and impact metrics, as well as tools for evaluating and validating security measures.
NVD provides a JSON data feed that contains information on newly added, modified or deleted vulnerabilities. In this blog post, we'll look at how to work with this JSON feed in JavaScript.
Fetching Data
The first thing we need to do is to get a JSON feed URL. NVD provides a JSON data feed which can be found at https://nvd.nist.gov/feeds/json/cve/1.1/nvdcve-1.1-recent.json.gz. You can find more details on formatting and usage of this URL in the official documentation.
Here's an example of how we can fetch data from this URL using the Fetch API:
fetch('https://nvd.nist.gov/feeds/json/cve/1.1/nvdcve-1.1-recent.json.gz')
.then(response => response.json())
.then(data => console.log(data))
In the above code, we're fetching the JSON data feed URL using the Fetch API. Once the data is fetched, we're calling the json()
method to parse the response as JSON and logging it to the console. This code will output the entire JSON feed in the console.
Filtering Data
The next step is to filter the JSON feed data based on certain criteria. We can use the filter()
method to filter the JSON data based on specific properties.
fetch('https://nvd.nist.gov/feeds/json/cve/1.1/nvdcve-1.1-recent.json.gz')
.then(response => response.json())
.then(data => {
const filteredData = data.CVE_Items.filter(item => {
return item.cve.description.description_data[0].value.includes('WordPress');
});
console.log(filteredData);
});
In this code, we're filtering the JSON data based on the cve.description.description_data
property which contains vulnerability descriptions. We're using the includes()
method to match the vulnerability description with the string 'WordPress'
.
Displaying Data
Finally, we need to display the filtered data in a meaningful way. We can use a template engine like Handlebars to create HTML templates dynamically.
fetch('https://nvd.nist.gov/feeds/json/cve/1.1/nvdcve-1.1-recent.json.gz')
.then(response => response.json())
.then(data => {
const filteredData = data.CVE_Items.filter(item => {
return item.cve.description.description_data[0].value.includes('WordPress');
});
const source = document.getElementById('template').innerHTML;
const template = Handlebars.compile(source);
const html = template({ data: filteredData });
document.getElementById('container').innerHTML = html;
});
In this code, we're using Handlebars to create an HTML template which will display the filtered data. We're fetching the HTML template from the DOM and then compiling it into a function using Handlebars.compile()
. We're then using the compiled function to generate HTML using the filtered data and inserting it into the container element with the ID container
.
Conclusion
In this blog post, we've looked at how to work with the NIST National Vulnerability Database JSON feed in JavaScript. We've fetched data from the feed, filtered it based on certain criteria, and displayed it using HTML templates. Now it's your turn to explore the feed and come up with your own ideas on how to use it in your projects.