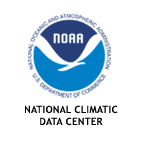
NOAA Climate Data
WeatherHow to Use NOAA CDO API to Retrieve Weather Data?
Are you looking for a reliable source of weather data for your project? NOAA CDO (National Oceanic and Atmospheric Administration Climate Data Online) API provides access to a wide range of weather-related information from various sources. In this blog post, we will discuss how to retrieve weather data using NOAA CDO API.
Requirements
To use the NOAA CDO API, you need to have an API token. You can sign up for a free account on their website https://www.ncdc.noaa.gov/cdo-web/webservices/v2, and get an API token.
Steps to Retrieve Weather Data using NOOA CDO API
Here are the steps to retrieve weather data using NOAA CDO API:
Step 1: Set up your JavaScript project
Create a new JavaScript project, and then install the fetch library using npm or yarn:
npm install node-fetch
Step 2: Set up your fetch request
To retrieve data from NOAA CDO API, you need to create a fetch request and set up the API endpoint, query parameters, and headers.
const fetch = require('node-fetch');
const apiEndpoint = 'https://www.ncdc.noaa.gov/cdo-web/webservices/v2';
const apiKey = 'YOUR_API_KEY';
const header = {
'Content-Type': 'application/json',
'token': apiKey,
};
const getRequest = async (url) => {
const response = await fetch(url, {
method: 'GET',
headers: header,
});
return response.json();
};
Step 3: Retrieve Weather Data for a Location
To retrieve weather data for a specific location, you need to set up the API endpoint, which provides various query parameters. For example, you can retrieve data for a specific station and date range. The following method retrieves all the weather data for a station within a date range:
async function getWeatherData(stationID, startDate, endDate) {
const url = `${apiEndpoint}/data?datasetid=GHCND&stationid=${stationID}&startdate=${startDate}&enddate=${endDate}`;
const data = await getRequest(url);
return data;
}
Step 4: Analyze the Returned Data
The returned data is in JSON format, which you can manipulate and analyze to extract the relevant data for your project. For example, you can retrieve the temperature data from the JSON object:
async function getTemperature(stationID, startDate, endDate) {
const url = `${apiEndpoint}/data?datasetid=GHCND&stationid=${stationID}&startdate=${startDate}&enddate=${endDate}`;
const data = await getRequest(url);
const temperatureData = data.results.map((i) => {
return {
date: i.date,
temperature: i.value,
};
});
return temperatureData;
}
Conclusion
NOAA CDO API provides a wide range of weather-related data, which you can use for various projects. With the above steps, you can retrieve weather data using JavaScript and analyze the returned data to extract the relevant information.