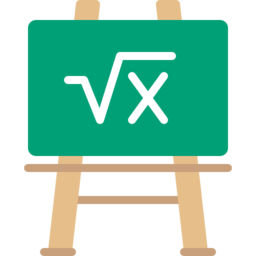
Numbers API
Open DataExploring Math.tools API with JavaScript
Math.tools provides a free and open API for developers to use in their projects. In this blog post, we'll explore some of the available endpoints and provide example code in JavaScript.
Getting Started
Before we begin, you'll need an API key. You can sign up for one for free on the Math.tools API documentation page.
To use the API in your JavaScript code, you will need to make HTTP requests to the API's endpoints using a library like Axios or Fetch.
Example Code
Here is an example of how to make a request to the sqrt
endpoint using Axios:
const axios = require('axios');
const BASE_URL = 'https://math.tools/api/numbers';
const API_KEY = '<insert your API key here>';
const number = 16;
axios.get(`${BASE_URL}/sqrt/${number}?apikey=${API_KEY}`)
.then(response => {
console.log(response.data.result);
})
.catch(error => {
console.log(error.response.data.error);
});
Here is the same request using Fetch:
const BASE_URL = 'https://math.tools/api/numbers';
const API_KEY = '<insert your API key here>';
const number = 16;
fetch(`${BASE_URL}/sqrt/${number}?apikey=${API_KEY}`)
.then(response => response.json())
.then(json => {
console.log(json.result);
})
.catch(error => {
console.log(error.message);
});
Note that in both examples, we need to include our API key in the request URL as a query parameter.
Available Endpoints
sqrt
This endpoint returns the square root of a number.
Example Request:
GET /sqrt/16?apikey=<your api key>
Example Response:
{
"success": true,
"result": 4
}
log
This endpoint returns the natural logarithm of a number.
Example Request:
GET /log/10?apikey=<your api key>
Example Response:
{
"success": true,
"result": 2.302585092994046
}
exp
This endpoint returns the exponential of a number.
Example Request:
GET /exp/2?apikey=<your api key>
Example Response:
{
"success": true,
"result": 7.3890560989306495
}
sin
This endpoint returns the sine of an angle in radians.
Example Request:
GET /sin/0.5?apikey=<your api key>
Example Response:
{
"success": true,
"result": 0.479425538604203
}
cos
This endpoint returns the cosine of an angle in radians.
Example Request:
GET /cos/0.5?apikey=<your api key>
Example Response:
{
"success": true,
"result": 0.8775825618903728
}
tan
This endpoint returns the tangent of an angle in radians.
Example Request:
GET /tan/0.5?apikey=<your api key>
Example Response:
{
"success": true,
"result": 0.5463024898437905
}
Conclusion
In this blog post, we've explored some of the available endpoints of the Math.tools API and provided example code in JavaScript using Axios and Fetch. With these tools, you can quickly and easily integrate mathematical calculations into your web applications.