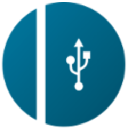
ODWeather
WeatherExploring the OceanDrivers API with JavaScript
The OceanDrivers API provides access to oceanographic and meteorological data collected from various sources around the world. In this article, we will explore some basic examples of how to use the API with JavaScript.
Getting Started
Firstly, you will need an API key to authenticate your requests. You can obtain a key by registering on the OceanDrivers website. Once you have your key, you can start making requests to the API endpoints.
To get the latest sea surface temperature data for a specific location, you can use the following JavaScript code:
const API_KEY = 'your-api-key';
const URL = 'http://api.oceandrivers.com/v1.0/getSeaSurfaceTemp';
const lat = 41.38;
const lng = 2.17;
const params = new URLSearchParams({
lat: lat,
lng: lng,
apiKey: API_KEY
});
fetch(`${URL}?${params}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we create a new URLSearchParams
object to store our request parameters. We then use the fetch
function to make a GET request to the API endpoint. The response is then converted to JSON format and logged to the console.
More Examples
Wind Forecast
To get the wind forecast data for a specific location and time period, you can use the following code:
const API_KEY = 'your-api-key';
const URL = 'http://api.oceandrivers.com/v1.0/getWindForecast';
const lat = 41.38;
const lng = 2.17;
const initDate = '2022-01-15T06:00:00Z';
const endDate = '2022-01-16T06:00:00Z';
const params = new URLSearchParams({
lat: lat,
lng: lng,
initDate: initDate,
endDate: endDate,
apiKey: API_KEY
});
fetch(`${URL}?${params}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Wave Forecast
To get the wave forecast data for a specific location and time period, you can use the following code:
const API_KEY = 'your-api-key';
const URL = 'http://api.oceandrivers.com/v1.0/getWaveForecast';
const lat = 41.38;
const lng = 2.17;
const initDate = '2022-01-15T06:00:00Z';
const endDate = '2022-01-16T06:00:00Z';
const params = new URLSearchParams({
lat: lat,
lng: lng,
initDate: initDate,
endDate: endDate,
apiKey: API_KEY
});
fetch(`${URL}?${params}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Weather Forecast
To get the weather forecast data for a specific location and time period, you can use the following code:
const API_KEY = 'your-api-key';
const URL = 'http://api.oceandrivers.com/v1.0/getWeatherForecast';
const lat = 41.38;
const lng = 2.17;
const initDate = '2022-01-15T06:00:00Z';
const endDate = '2022-01-16T06:00:00Z';
const params = new URLSearchParams({
lat: lat,
lng: lng,
initDate: initDate,
endDate: endDate,
apiKey: API_KEY
});
fetch(`${URL}?${params}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
The OceanDrivers API provides a range of useful data for oceanographic and meteorological research. By using JavaScript to interact with the API endpoints, you can easily access and analyze this data in your own web applications. The examples provided above are just the tip of the iceberg of what can be achieved with the OceanDrivers API.