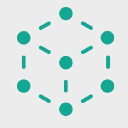
Open Government, Italy
GovernmentItaly Government Open Data. Italy government is sharing its data on economy, finances, education, culture, sports, power, environment, government, public sector, health, justice, legal systems, public safety, regions, cities, population, society, science, technology and transport
📚 Documentation & Examples
Everything you need to integrate with Open Government, Italy
🚀 Quick Start Examples
// Open Government, Italy API Example
const response = await fetch('https://www.dati.gov.it/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Public API Docs - www.dati.gov.it
Dati.gov.it is Italy's public data portal, where government agencies provide free access to their datasets. Dati.gov.it's API provides programmatic access to this data, allowing developers to integrate public data into their own applications.
API Overview
The API for dati.gov.it is RESTful and provides data in JSON format. The base URL for the API is https://www.dati.gov.it/api/3/action/
.
Authentication
Dati.gov.it does not require authentication to access its public data.
API Examples in JavaScript
Example 1: Search Datasets
const searchDatasets = async (query) => {
const url = `https://www.dati.gov.it/api/3/action/package_search?q=${query}`;
try {
const response = await fetch(url);
const data = await response.json();
console.log(data.result.results);
} catch (error) {
console.error(error);
}
};
searchDatasets("healthcare");
This function searches for datasets with given query string.
Example 2: Get Dataset by ID
const getDatasetById = async (id) => {
const url = `https://www.dati.gov.it/api/3/action/package_show?id=${id}`;
try {
const response = await fetch(url);
const data = await response.json();
console.log(data.result);
} catch (error) {
console.error(error);
}
};
getDatasetById("e6f5d835-0083-4be4-bd22-1b0a71fc9f4e");
This function retrieves the dataset metadata by providing ID of the dataset.
Example 3: Get Resource by ID
const getResourceById = async (id) => {
const url = `https://www.dati.gov.it/api/3/action/resource_show?id=${id}`;
try {
const response = await fetch(url);
const data = await response.json();
console.log(data.result);
} catch (error) {
console.error(error);
}
};
getResourceById("d0b674b9-6293-4bc5-a6c4-78b772910b5e");
This function retrieves the resource metadata by providing ID of the resource.
Conclusion
Dati.gov.it's API provides easy access to public data that can be leveraged to build powerful applications. With the examples given above, developers can get started with integrating it into their own projects.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes