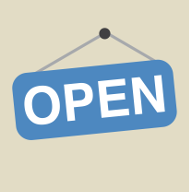
Open Library API
Open DataOpen Library has a RESTful API, best used to link into Open Library data in JSON, YAML and RDF/XML. Open Library has developed a suite of APIs to help developers get up and running with our data. We encourage interested developers to join the ol-tech mailing list to stay up-to-date with the latest news, or dive in with our own development team at our bug tracker or our GitHub source code repository.
📚 Documentation & Examples
Everything you need to integrate with Open Library API
🚀 Quick Start Examples
// Open Library API API Example
const response = await fetch('https://openlibrary.org/developers/api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Introduction to Open Library API
Open Library is a project of the Internet Archive, designed to create a web page for every book ever published. Open Library allows users to search for books, view book information and covers, and create their own reading lists. Open Library also provides an API that developers can use to access book information programmatically.
This API provides various endpoints to fetch book data based on different criteria. Here, we will look at some examples of using the Open Library API in Javascript.
Example Code
Example 1: Fetching book details by ISBN
const isbn = '0451526538';
const apiUrl = `https://openlibrary.org/api/books?bibkeys=ISBN:${isbn}&format=json`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
const book = data[`ISBN:${isbn}`];
console.log(book.title);
console.log(book.authors);
console.log(book.publish_date);
})
.catch(error => console.log(error));
In the above example, we are fetching book information using an ISBN number. We first construct the API URL and make a GET request using fetch()
. We then extract the required fields from the JSON response.
Example 2: Searching books by author name
const authorName = 'J. K. Rowling';
const apiUrl = `https://openlibrary.org/search.json?author=${encodeURIComponent(authorName)}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
const authorBooks = data.docs.filter(doc => doc.author_name.includes(authorName));
console.log(authorBooks);
})
.catch(error => console.log(error));
In the above example, we first encode the author name using encodeURIComponent()
and construct the API URL with the encoded name. We then make a GET request to the search.json
endpoint and filter the book results based on the author name.
Example 3: Fetching book covers
const bookUrl = 'https://openlibrary.org/works/OL45883W';
const apiUrl = `${bookUrl}.json`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
const covers = data.cover;
if (covers) {
console.log(covers[0]);
} else {
console.log('No cover available.');
}
})
.catch(error => console.log(error));
In the above example, we are fetching the cover images of a book given its URL. We append .json
to the URL and make a GET request to fetch the book data. We then extract the cover images from the response.
Conclusion
This was just a brief introduction to the Open Library API and some examples of using it in Javascript. The API provides a range of endpoints to access book data, which can be used in various use cases. Refer to the Open Library API documentation for more information.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes