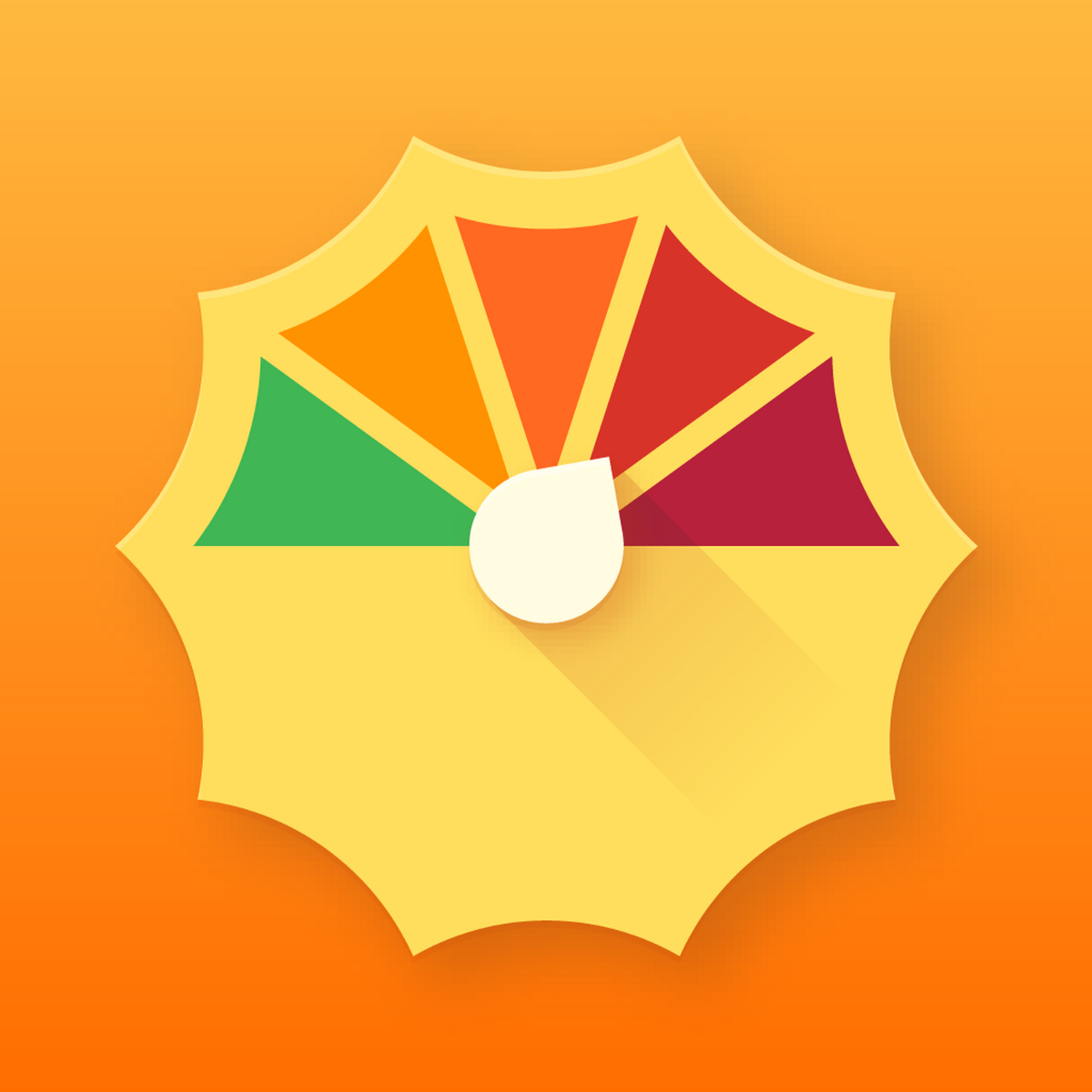
OpenUV
WeatherGetting Started with OpenUV API
OpenUV API is a public API that provides UV index forecast data for any location on the planet. In this blog post, we will cover how to use the OpenUV API to get UV index forecast data for any location using JavaScript.
API Key
Before you can use the OpenUV API, you will need to sign up for an API key. To sign up, go to the OpenUV website and create an account. Once you have an account, you will be able to generate an API key from your dashboard.
API Endpoints
OpenUV API provides a number of endpoints that can be used to get UV index forecast data. The following are some of the endpoints that are available:
/api/v1/uv
- Returns the current UV index data for a given location./api/v1/uv_history
- Returns the UV index data history for a given location./api/v1/forecast
- Returns the UV index forecast data for a given location.
For the purpose of this blog post, we will focus on how to use the /api/v1/uv
endpoint to get current UV index data for a given location.
Code Examples
The following JavaScript code examples demonstrate how to use the OpenUV API to get UV index forecast data for a given location:
const apiKey = 'your_api_key';
const latitude = 37.7749;
const longitude = -122.4194;
fetch(`https://api.openuv.io/api/v1/uv?lat=${latitude}&lng=${longitude}`, {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'x-access-token': apiKey
},
})
.then(res => res.json())
.then(data => console.log(data))
.catch(err => console.log(err));
In the above example, we first define the API key, latitude, and longitude for the location that we want to get UV index data for. We then use the fetch
function to send a GET request to the /api/v1/uv
endpoint with the latitude and longitude as query parameters. We also include the API key as a header in the request.
Once the request is successful, we parse the response JSON data and log it to the console.
Conclusion
In this blog post, we covered how to use the OpenUV API to get UV index forecast data for any location using JavaScript. The API provides a number of endpoints that can be used to get UV index data, and we demonstrated how to retrieve current UV index data using the /api/v1/uv
endpoint.