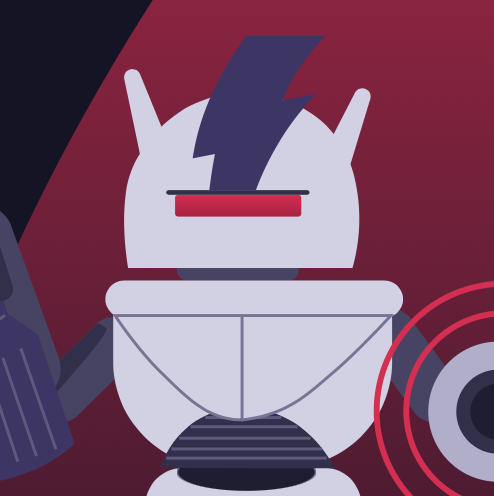
Overwolf API
Games & ComicsEasily create apps for PC gamers. Overlay, real-time game events, monetisation, auto patching, Anti-Cheat compatibility and all that good stuff. Create a desktop app with overlay capabilities, using just HTML and JS. Your own desktop app, desktop icon, auto updates, anti-cheat compatibility and the works... we do it all for you. Get access to real-time game events for leading games and make your app as if it were integrated directly with the game itself. Monetize your app and make money by showing forgivable ads (= ads on loading screens, when gamers don’t have anything better to do anyway). It’s easier than implementing AdSense, not affected by ad blockers, not obtrusive and our team will optimise the experience with you. Community is power and a driver for retention. Give your community tools to create valuable in-game content for your game with the Overwolf SDK.
📚 Documentation & Examples
Everything you need to integrate with Overwolf API
🚀 Quick Start Examples
// Overwolf API API Example
const response = await fetch('https://overwolf.github.io/docs/api/overwolf-api-overview', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
An Overview of the Overwolf API
Overwolf is a platform that allows developers to create apps that run on top of existing games. It provides a range of APIs that allow developers to access a variety of features of the games and the Overwolf platform.
The Overwolf API is a collection of APIs that enable developers to create apps for games. These APIs provide access to game events and data, as well as platform-specific features like in-game overlays, database storage, and more.
Here are a few example code snippets in JavaScript that demonstrate how to use some of the APIs available in the Overwolf API:
Getting the current game ID
overwolf.games.getRunningGameInfo(function(gameInfo) {
var gameId = gameInfo.gameId;
console.log("Current game ID:", gameId);
});
This code uses the overwolf.games.getRunningGameInfo
API to get information about the currently running game. The gameId
property of the gameInfo
object is then used to get the ID of the game.
Creating an in-game overlay
overwolf.windows.obtainDeclaredWindow("MyOverlay", function(result) {
if (result.status === "success") {
overwolf.windows.restore(result.window.id, function(result) {
console.log("Overlay restored:", result.window.id);
});
}
});
This code uses the overwolf.windows.obtainDeclaredWindow
API to get a reference to an overlay window called "MyOverlay". If the window exists, the code then uses overwolf.windows.restore
to show the overlay.
Saving data to the Overwolf database
var dataObj = { key1: "value1", key2: "value2" };
overwolf.profile.getCurrentUser(function(userInfo) {
overwolf.profile.setUserData(userInfo.username, "myData", dataObj, function(result) {
console.log("Data saved:", result);
});
});
This code uses the overwolf.profile.setUserData
API to save a JavaScript object to the Overwolf database under a key named "myData". The getCurrentUser
API is used to get the username of the currently logged-in user, which is used as the database name.
Conclusion
The Overwolf API provides a powerful set of APIs that allow developers to easily create apps for games. These examples give a taste of what's possible with the Overwolf API and should help get started building your own game apps. Check out the full API documentation for more information on how to use the various APIs available.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes