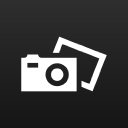
Pixabay
PhotographyIntroduction to Pixabay API
Pixabay is a platform that offers free images and videos. The platform, among other features, provides APIs that developers can use to access images or videos. The API provides many different parameters to filter images based on specific requirements, including image type, size, and orientation. In this article, we will explore Pixabay API and provide some example code in JavaScript.
API Key
Before we start using the API, we need to obtain an API key. The API key is required for authentication in all requests made to the Pixabay API. The API key can be obtained by creating an account and then navigating to the API documentation page.
Using the API
Let's now examine how we can use the API to retrieve images. In the example below, the API is used to retrieve five images related to the keyword "nature".
const axios = require('axios');
const apiKey = 'YOUR_API_KEY';
axios.get(`https://pixabay.com/api/?key=${apiKey}&q=nature&per_page=5`)
.then(function (response) {
const data = response.data.hits;
data.forEach(function (item) {
console.log(item.previewURL);
});
})
.catch(function (error) {
console.log(error);
});
The code above uses the axios library, which is commonly used to handle HTTP requests. We start by defining the API key we obtained earlier in the apiKey
constant. We then use axios.get
to send a GET request to the Pixabay API. In the GET request URL, we specify the apiKey
, the q
parameter for the search query (nature
in this case), and the per_page
parameter to limit the number of images returned to 5. In the response handler function, we extract the image preview URLs from the hits
object in the response.
Filtering Results
The API provides various parameters to filter the results based on our specific requirements. We can filter by image types, categories, colors, and many more attributes.
axios.get(`https://pixabay.com/api/?key=${apiKey}&q=flower&per_page=5&safesearch=true&order=popular`)
.then(function (response) {
const data = response.data.hits;
data.forEach(function (item) {
console.log(item.previewURL);
});
})
.catch(function (error) {
console.log(error);
});
In the example above, we have added two additional parameters to filter the results. The safeSearch
parameter is set to true
, which ensures that the results will not display any adult content. The order
parameter is set to popular
, which ensures that the results are sorted by their popularity, ensuring that the most popular images appear on top.
Conclusion
In summary, we can use the Pixabay API to retrieve images and videos. The API provides many parameters to filter the results based on our specific requirements. We can use JavaScript to send HTTP requests to the API and handle the response to display the desired results.