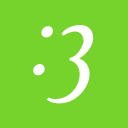
PlaceKitten
PhotographyUsing the PlaceKitten API in JavaScript
The PlaceKitten API is a public API that offers a simple way to generate placeholder kitten images for your projects. In this article, we will explore how to use this API in JavaScript to generate kitten images.
Getting started with the PlaceKitten API
To generate a kitten image using the PlaceKitten API, all you need to do is to make an HTTP request to the API. The API then returns a kitten image in response.
Here is a simple example of how to make an HTTP request to the PlaceKitten API using fetch
in JavaScript:
fetch('https://placekitten.com/200/300')
.then(response => {
// do something with the response
})
In this example, we are making a request to the https://placekitten.com/200/300
URL, which generates a kitten image that is 200 pixels wide and 300 pixels tall. The fetch
function returns a Promise, which resolves when the server returns a response.
Handling the response
Once we get the response from the API, we can process it in different ways. For example, we can use the Blob
API to convert the response to an image that we can display on a web page.
Here is an example of how to display a kitten image in an HTML element:
<img id="kitten" src="">
const kittenImg = document.getElementById('kitten');
fetch('https://placekitten.com/200/300')
.then(response => response.blob())
.then(blob => {
kittenImg.src = URL.createObjectURL(blob);
})
In this example, we are creating an img
element with an id
of kitten
. We then make a request to the API and use the blob
method to convert the response to a Blob
object. Finally, we set the src
attribute of the img
element to a URL that points to the Blob
object.
Conclusion
In this article, we learned how to use the PlaceKitten API in JavaScript to generate placeholder kitten images. We explored how to make an HTTP request to the API, handle the response, and display the kitten image on a web page. With this knowledge, you can now use the PlaceKitten API in your own projects to add some extra cuteness.