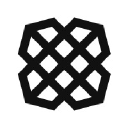
Plaid
FinanceConnecting with users' bank accounts has never been easier, thanks to our API that allows developers to access transaction data seamlessly. With this robust solution, applications can offer personalized financial insights, facilitate payment processing, and enhance budgeting tools. By integrating with users’ banking information, businesses can foster a more engaging and tailored experience, enabling users to track their spending, manage finances effortlessly, and make informed decisions. The API is built with security and ease of use in mind, ensuring that sensitive financial data is handled with the utmost care while providing unmatched access to vital transaction information.
The benefits of using this API are numerous and impactful:
- Streamlined user onboarding by directly linking bank accounts.
- Real-time transaction data retrieval for up-to-date financial insights.
- Enhanced security measures that protect user data.
- Customizable features to fit various business models and user needs.
- Improved user engagement through actionable financial recommendations.
Here’s a JavaScript code example for calling the API to retrieve transaction data:
const plaid = require('plaid');
const client = new plaid.Client({
clientID: 'YOUR_CLIENT_ID',
secret: 'YOUR_SECRET',
env: plaid.environments.sandbox,
});
client.getTransactions('ACCESS_TOKEN', '2023-01-01', '2023-01-31', {}, (err, response) => {
if (err) {
console.error('Error fetching transactions:', err);
} else {
console.log('Transaction data:', response.transactions);
}
});