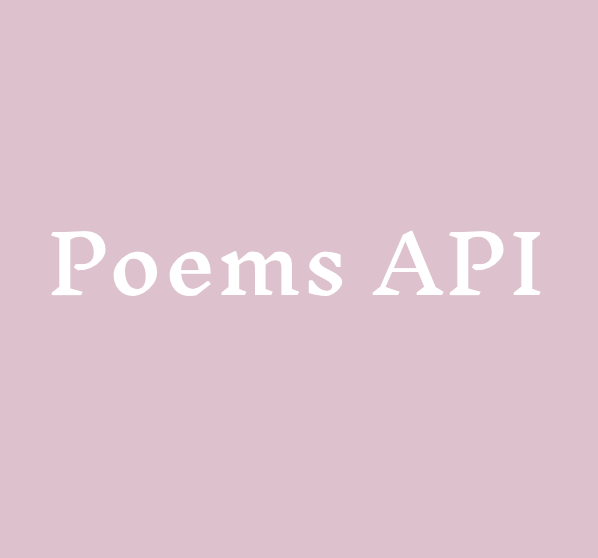
Poems
Books#Using the Poems.one API with JavaScript
Are you a lover of poetry? Do you want to get access to a collection of poems programmatically? Poems.one provides a public API that allows developers to fetch poems from their database.
##Requirements
To use the Poems.one API, you need an API key. You can get it by visiting the official Poems.one website [https://poems.one/] and creating an account. Once you have your API key, you are ready to use the API.
##API Endpoints
The Poems.one API provides two endpoints for you to fetch poems. These are:
- /api/poem/
- /api/random/
##Fetching a random poem
To fetch a random poem, you can use the /api/random/ endpoint.
fetch("https://poems.one/api/random", {
headers: {
"X-API-KEY": "YOUR_API_KEY"
}
})
.then(response => {
if(!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(json => console.log(json))
.catch(error => console.log('Error:', error));
##Fetching a poem by ID
To fetch a poem by ID, you can use the /api/poem/ endpoint.
fetch("https://poems.one/api/poem/1", {
headers: {
"X-API-KEY": "YOUR_API_KEY"
}
})
.then(response => {
if(!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(json => console.log(json))
.catch(error => console.log('Error:', error));
In the above examples, replace "YOUR_API_KEY" with your actual API key.
##Conclusion
The Poems.one API is a simple, yet useful tool for developers who want to fetch poems programmatically. With a few lines of JavaScript, you can access a large collection of poems and use them in your applications or projects.