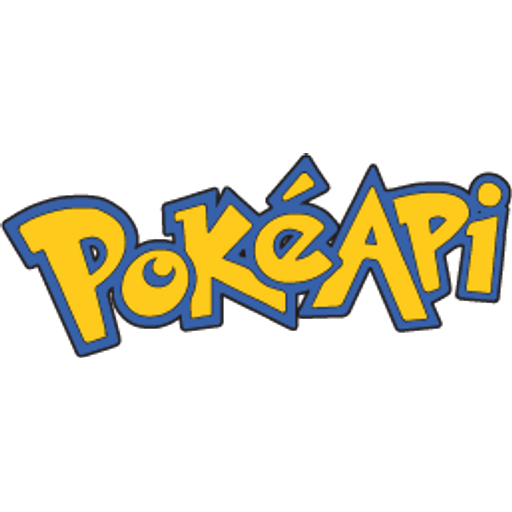
Pokéapi
Games & ComicsIntroduction to PokeAPI
PokeAPI is a free-to-use public API that gives you access to information about Pokemon characters. You can use this API to retrieve data about Pokemon species, abilities, moves, and much more.
In this blog post, we will guide you through how to use PokeAPI in JavaScript. We will start by exploring the available endpoints and then show you all possible API example code that you can use in your project.
Available Endpoints in PokeAPI
PokeAPI provides various endpoints to retrieve data about Pokemon characters. Here are some of the most commonly used endpoints in PokeAPI:
https://pokeapi.co/api/v2/pokemon/{id or name}
- Retrieves data about a specific Pokemon character.https://pokeapi.co/api/v2/pokemon-species/{id or name}
- Retrieves data about a specific Pokemon species.https://pokeapi.co/api/v2/move/{id or name}
- Retrieves data about a specific Pokemon move.https://pokeapi.co/api/v2/ability/{id or name}
- Retrieves data about a specific Pokemon ability.https://pokeapi.co/api/v2/type/{id or name}
- Retrieves data about a specific Pokemon type.https://pokeapi.co/api/v2/evolution-chain/{id}
- Retrieves data about a specific Pokemon evolution chain.
To use these endpoints, you need to send HTTP requests to the PokeAPI server and receive JSON responses that contain the desired data.
API Example Code in JavaScript
Let's take a look at some example code in JavaScript that uses PokeAPI:
Retrieve Data About a Specific Pokemon Character
const pokemonName = "pikachu";
fetch(`https://pokeapi.co/api/v2/pokemon/${pokemonName}`)
.then(response => response.json())
.then(data => console.log(data));
This code sends an HTTP GET request to retrieve data about the "pikachu" Pokemon character and logs the retrieved data to the console.
Retrieve Data About a Specific Pokemon Species
const speciesId = 25; // Pikachu's species ID
fetch(`https://pokeapi.co/api/v2/pokemon-species/${speciesId}`)
.then(response => response.json())
.then(data => console.log(data));
This code sends an HTTP GET request to retrieve data about the "pikachu" Pokemon species and logs the retrieved data to the console.
Retrieve Data About a Specific Pokemon Move
const moveName = "thunderbolt";
fetch(`https://pokeapi.co/api/v2/move/${moveName}`)
.then(response => response.json())
.then(data => console.log(data));
This code sends an HTTP GET request to retrieve data about the "thunderbolt" Pokemon move and logs the retrieved data to the console.
Retrieve Data About a Specific Pokemon Ability
const abilityName = "static";
fetch(`https://pokeapi.co/api/v2/ability/${abilityName}`)
.then(response => response.json())
.then(data => console.log(data));
This code sends an HTTP GET request to retrieve data about the "static" Pokemon ability and logs the retrieved data to the console.
Retrieve Data About a Specific Pokemon Type
const typeName = "electric";
fetch(`https://pokeapi.co/api/v2/type/${typeName}`)
.then(response => response.json())
.then(data => console.log(data));
This code sends an HTTP GET request to retrieve data about the "electric" Pokemon type and logs the retrieved data to the console.
Retrieve Data About a Specific Pokemon Evolution Chain
const chainId = 4; // Pikachu's evolution chain ID
fetch(`https://pokeapi.co/api/v2/evolution-chain/${chainId}`)
.then(response => response.json())
.then(data => console.log(data));
This code sends an HTTP GET request to retrieve data about the "pikachu" Pokemon evolution chain and logs the retrieved data to the console.
Conclusion
In this blog post, we have explored the PokeAPI and showed you all possible API example code in JavaScript. You can use these examples to retrieve data about Pokemon characters, species, moves, abilities, types, and evolution chains.
With this knowledge, you can start building your own Pokemon-related project using the PokeAPI. Happy coding!