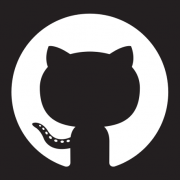
Programming Quotes API
Open Data'---
Explore the Programming Quotes API
Programming Quotes API is a collection of programming-related quotes from various people in the technology field. This API is publicly available and can be used for educational purposes.
Getting Started
To start using the Programming Quotes API, all you need is a web browser or any programming language that can consume an HTTP API. You can access the API by sending a GET request to http://programming-quotes-api.herokuapp.com/quotes/random
. This will return a random programming quote in JSON format.
fetch('http://programming-quotes-api.herokuapp.com/quotes/random')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
This code uses the fetch()
function to send a GET request to the Programming Quotes API. The response is then converted to JSON format using the .json()
function, and the data is logged to the console.
API Reference
The Programming Quotes API provides the following endpoints:
GET /quotes/random
This endpoint returns a random programming quote.
fetch('http://programming-quotes-api.herokuapp.com/quotes/random')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
GET /quotes/lang/{language}
This endpoint returns a random programming quote in the specified language.
fetch('http://programming-quotes-api.herokuapp.com/quotes/lang/en')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
GET /quotes/author/{author}
This endpoint returns a random programming quote from the specified author.
fetch('http://programming-quotes-api.herokuapp.com/quotes/author/Steve%20Jobs')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
GET /quotes/search/{query}
This endpoint searches for a programming quote that contains the specified query in its text or author name.
fetch('http://programming-quotes-api.herokuapp.com/quotes/search/java')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
Conclusion
The Programming Quotes API is a great resource for anyone interested in programming. With its simple and easy-to-use API, you can quickly retrieve programming-related quotes for educational or entertainment purposes.