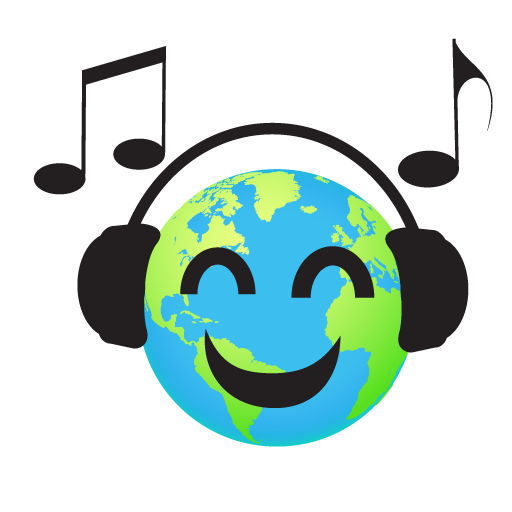
Radio Browser
Open DataHow to Use the Radio-Browser Public API
Radio-Browser is a public API that allows you to search for internet radio stations from all over the world. This API provides various endpoints that let you search for stations by region, genre, language, and much more. In this tutorial, we will walk you through how to use the Radio-Browser public API using JavaScript.
Getting Started
To get started, you will need to access the Radio-Browser API documentation available at http://www.radio-browser.info/webservice. Here, you will find all the documentation and endpoints available for use.
Example Code
We will now display a few examples of how to use the Radio-Browser API using JavaScript.
Example 1 - Search for Radio Stations by Country
let country = "United States"; // country to search for
let url = `http://www.radio-browser.info/webservice/json/stations/bycountryexact/${country}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data); // display returned data in console
})
.catch(error => console.error(error));
Example 2 - Search for Radio Stations by Language
let language = "English"; // language to search for
let url = `http://www.radio-browser.info/webservice/json/stations/bylanguage/${language}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error(error));
Example 3 - Search for Radio Stations by Tag
let tag = "pop"; // tag to search for
let url = `http://www.radio-browser.info/webservice/json/stations/bytag/${tag}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error(error));
Conclusion
The Radio-Browser public API is a useful tool for developers looking to build applications that use radio stations. It provides various endpoints that can help you find stations by country, language, genre, and more. In this tutorial, we walked you through how to use the Radio-Browser API using JavaScript. We hope that you found this tutorial helpful and that it has inspired you to build exciting applications.