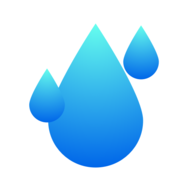
RainViewer
WeatherRainViewer Public API Documentation
RainViewer is a weather platform that provides real-time and historical radar data, forecasts, and alerts. They have a publicly accessible API that developers can integrate into their applications for weather-related information. In this article, we will explore RainViewer's Public API Documentation and provide examples in JavaScript.
API Access Key
Before using their API, you need to sign up for a free account on their website and generate your API key. You can do it here: https://www.rainviewer.com/data-api.html#account.
JavaScript Example
In this example, we will use the RainViewer API to retrieve data about precipitation.
- First, create an HTML file and include the following code snippet in the
<head>
section:
<script src="https://code.jquery.com/jquery-3.3.1.min.js"></script>
This code adds the jQuery library to your HTML file that will help to make API requests.
- Then, create a JavaScript file and include the following code snippet:
const apiKey = "<YOUR_API_KEY>";
const baseUrl = "https://api.rainviewer.com/public/weather-maps";
function getRadarData() {
const requestUrl = `${baseUrl}/precipitation/nowcast?product=radar&apikey=${apiKey}`;
$.get(requestUrl, function (data) {
console.log(data);
});
}
getRadarData();
In this code, we declare apiKey
and baseUrl
variables that will be used to construct the API request URL. Then, we define the getRadarData
function that constructs the request URL for real-time precipitation data and makes an AJAX request using the $.get
method. Finally, we call the getRadarData
function.
- Now, open the HTML file in your browser and check the console for the output.
Conclusion
RainViewer Public API provides real-time and historical weather information that you can integrate into your applications easily. In this post, we explored how to retrieve real-time precipitation data using the JavaScript programming language. You can find more information about their API features and endpoints on their documentation page: https://www.rainviewer.com/api.html.