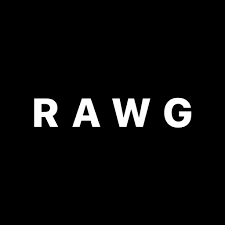
RAWG API
Games & ComicsOpen video game database. Find games by platform, genre, company or find game developers, stores, publishers and many more.
📚 Documentation & Examples
Everything you need to integrate with RAWG API
🚀 Quick Start Examples
// RAWG API API Example
const response = await fetch('https://rawg.io/apidocs', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Discover the World of Gaming with RAWG API
Are you a gaming enthusiast, looking for an easy way to access gaming data? Look no further, because the RAWG API might just be what you need.
Introduction
RAWG is a gaming database that contains information on over 500,000 games, including details such as release dates, ratings, genres, developers, platforms and more. RAWG API offers public access to all this data, allowing developers to integrate gaming information into their own applications.
Getting Started
To start using the RAWG API, you'll need an API key. You can obtain this by signing up on their website. Once you have your API key, you can begin making requests.
Example Code
Below is an example code written in JavaScript that demonstrates how to make an API request to retrieve a list of games:
const apiKey = "your_api_key_here";
fetch(`https://api.rawg.io/api/games?key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this code snippet, we are using the fetch
function to make an API request to the RAWG endpoint (https://api.rawg.io/api/games
) and passing our API key as a query parameter (key
). Once the response is received, we are parsing the JSON data and logging it to the console.
Endpoints
The RAWG API provides various endpoints to retrieve different types of game data. Some of the commonly used endpoints are:
/games
: Returns a list of games/games/{id}
: Returns details of a specific game/genres
: Returns a list of genres/platforms
: Returns a list of platforms
Example Code
Here's another example code written in JavaScript that demonstrates how to make a request to retrieve details of a specific game:
const apiKey = "your_api_key_here";
const gameId = "3498"; // Replace with your desired game ID
fetch(`https://api.rawg.io/api/games/${gameId}?key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this code snippet, we are using the /games/{id}
endpoint to retrieve the details of a specific game by passing the game ID as a path parameter.
Conclusion
In this article, we discussed the RAWG API and demonstrated how to make API requests using JavaScript. With this API, developers can easily access gaming data from a vast database and create gaming applications that are feature-rich and engaging. So, start exploring the API and create your gaming integration today!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes