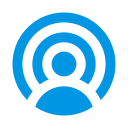
Remotive Job API
JobsUsing Remotive API Documentation with JavaScript
Remotive offers a public API which allows users to access job listings and company insights related to remote work. In this blog post, we will explore how to use Remotive's API Documentation with JavaScript.
API Key
Before we can start making API requests, we need to obtain an API key. To obtain an API key, follow these simple steps:
- Go to https://remotive.io/api-key.
- Enter your email address and click on the "Generate API Key" button.
- Check your email inbox for an email from Remotive containing your API key.
Making API requests with JavaScript
In order to make API requests with JavaScript, we can use the XMLHttpRequest object (XHR). The XHR object makes it easy to send HTTP requests to the Remotive API endpoint.
To send a GET request, we can create a new XMLHttpRequest object and call the open()
and send()
methods. Here's an example:
const endpoint = 'https://remotive.io/api/remote-jobs?category=software-dev';
const xhr = new XMLHttpRequest();
xhr.open('GET', endpoint, true);
xhr.send();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
const data = JSON.parse(xhr.responseText);
console.log(data.jobs);
} else {
console.log('Error: ' + xhr.status);
}
};
In the code above, we make a GET request to the Remotive API endpoint for remote software development jobs. We then listen for changes in the XHR object's state and, if the request is successful (status code 200), we log the job data to the console.
Conclusion
By following the steps outlined in this blog post, you should now be able to make API requests to the Remotive API endpoint using JavaScript. Be sure to check out the Remotive API Documentation for all available API endpoints and parameters. Happy coding!