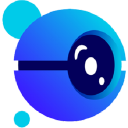
Rocketium Video API
VideoExploring Rocketium API
Rocketium offers a powerful RESTful API that makes it easy to add video and image editing functionality to your application. The API supports various functionalities like creating and rendering videos, adding text overlays, generating memes, etc.
Authentication
Rocketium's API requires an access token to authorize access to your account. You can obtain the token from the dashboard.
const api_key = 'your_api_key';
Creating a Video
To create a video, you need to first make a POST request to the /videos
endpoint with a JSON payload containing the video details.
const postData = {
title: "My Rocketium Video",
storyboard_id: 12345,
export_quality: "720p",
fps: 30,
custom_data: {
my_data: "123"
}
};
const options = {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${api_key}`
},
body: JSON.stringify(postData)
};
const response = await fetch('https://api.rocketium.com/v1/videos', options);
const video = await response.json();
Adding Text Overlay
You can add text overlays to your videos using the /videos/<video_id>/overlays
endpoint.
const postData = {
text: "Hello World",
font_type: "Arial",
font_size: 36,
horizontal_alignment: "center",
vertical_alignment: "middle",
color: "#FFFFFF",
background_color: "#000000",
start_time: "00:00:00.00",
end_time: "00:00:10.00"
};
const options = {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${api_key}`
},
body: JSON.stringify(postData)
};
const response = await fetch(`https://api.rocketium.com/v1/videos/${video.id}/overlays`, options);
const overlay = await response.json();
Generating a Meme
You can use Rocketium's meme API to generate memes containing custom text.
const postData = {
template_id: 123,
top_text: "First World Problems",
bottom_text: "My coffee is too hot",
font: "Arial",
font_size: 24,
background_color: "#FFFFFF",
font_color: "#000000"
};
const options = {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${api_key}`
},
body: JSON.stringify(postData)
};
const response = await fetch(`https://api.rocketium.com/v1/memes`, options);
const meme = await response.json();
Conclusion
Rocketium's API offers a variety of useful functionalities that can enhance your application's video and image editing capabilities. The examples provided above only scratch the surface of what's possible with this powerful API. To learn more, check out the official Rocketium API documentation at https://rocketium.com/api.