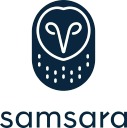
Samsara API
Open DataGetting Started with the Samsara API
Samsara is a platform that provides a suite of APIs for fleet management and industrial IoT applications. In this tutorial, we will be focusing on the Samsara API and how it can be used to interact with the platform.
Authentication
Before you can use the Samsara API, you need to obtain an API access token. You can do this by following the instructions on the Samsara API documentation.
Once you have your access token, you will need to provide it in the API requests. This can be done by setting the Authorization
header to Bearer <access_token>
.
Using the Samsara API
The Samsara API provides a range of endpoints for interacting with the platform. Here are some examples of how to use them in JavaScript:
Getting Vehicles
To retrieve a list of all vehicles on your Samsara account, use the GET /fleet/vehicles
endpoint:
const fetch = require("node-fetch");
const baseUrl = "https://api.samsara.com/v1";
const accessToken = "<your_access_token>";
const getVehicles = async () => {
const res = await fetch(`${baseUrl}/fleet/vehicles`, {
headers: { Authorization: `Bearer ${accessToken}` },
});
const data = await res.json();
console.log(data);
};
getVehicles();
Getting Assets
To retrieve a list of all assets on your Samsara account, use the GET /assets
endpoint:
const fetch = require("node-fetch");
const baseUrl = "https://api.samsara.com/v1";
const accessToken = "<your_access_token>";
const getAssets = async () => {
const res = await fetch(`${baseUrl}/assets`, {
headers: { Authorization: `Bearer ${accessToken}` },
});
const data = await res.json();
console.log(data);
};
getAssets();
Creating a Tag
To create a new tag on your Samsara account, use the POST /tags
endpoint:
const fetch = require("node-fetch");
const baseUrl = "https://api.samsara.com/v1";
const accessToken = "<your_access_token>";
const createTag = async () => {
const body = {
name: "My New Tag",
assets: ["123456", "789012"],
};
const res = await fetch(`${baseUrl}/tags`, {
method: "POST",
headers: { Authorization: `Bearer ${accessToken}` },
body: JSON.stringify(body),
});
const data = await res.json();
console.log(data);
};
createTag();
Conclusion
In this tutorial, we have covered the basics of using the Samsara API in JavaScript. The Samsara API provides a wealth of endpoints for managing vehicles, assets, and more. By using the examples provided, you should now have a good understanding of how to get started with the Samsara API.