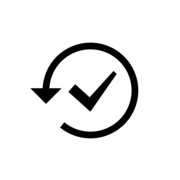
SecurityTrails
SecurityExploring SecurityTrails API Documentation
SecurityTrails API documentation provides developers access to a vast amount of historical and real-time data related to the security of internet assets. With the help of powerful API endpoints, developers can easily integrate SecurityTrails API into their applications and automate different security tasks.
Getting Started
Before getting into API examples, you should create an account on SecurityTrails website. After signing up, you will get your API key which you can use to authenticate all API requests.
API Examples in JavaScript
Below are some examples of how to use SecurityTrails API using JavaScript.
Domain IP History
const axios = require('axios');
const apiKey = 'YOUR_API_KEY_HERE';
axios.get(`https://api.securitytrails.com/v1/domain/YOUR_DOMAIN/ip-history`, {
headers: {
'APIKEY': apiKey
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
This API endpoint provides you with a historical list of all IP addresses associated with a domain. Replace YOUR_DOMAIN
with the target domain to get the results.
Reverse IP Lookup
const axios = require('axios');
const apiKey = 'YOUR_API_KEY_HERE';
const ipAddress = 'TARGET_IP_ADDRESS';
axios.get(`https://api.securitytrails.com/v1/ips/${ipAddress}/associated`, {
headers: {
'APIKEY': apiKey
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
This API endpoint helps you to find all the domains associated with a particular IP address. Replace TARGET_IP_ADDRESS
with the target IP address to get the results.
WHOIS Lookup
const axios = require('axios');
const apiKey = 'YOUR_API_KEY_HERE';
const domain = 'TARGET_DOMAIN';
axios.get(`https://api.securitytrails.com/v1/domain/${domain}/whois`, {
headers: {
'APIKEY': apiKey
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
This API endpoint provides you with the complete WHOIS record for a particular domain. Replace TARGET_DOMAIN
with the target domain to get the results.
Conclusion
In conclusion, SecurityTrails API documentation offers a reliable way to perform security tasks and gather historical data related to internet assets. With easy-to-use API endpoints, getting access to the data is fast and straightforward. This blog post provides you with JavaScript code snippets that demonstrate how to use SecurityTrails API to retrieve data. For more detailed documentation, visit the SecurityTrails API docs.