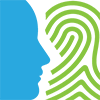
Skybiometry
Machine LearningBuilding Applications with SkyBiometry Public API
SkyBiometry provides a facial detection and recognition API that allows developers to create intelligent applications. To begin, you must sign up for an account at SkyBiometry. Once you have an account, you will receive a Client ID and Client Secret. These credentials will enable you to access the API endpoints.
API Endpoints
Detect Faces
Detect Faces endpoint analyzes an image and returns with the facial features of all the persons detected in the image.
POST https://api.skybiometry.com/fc/faces/detect
Example Code in JavaScript
const axios = require("axios");
const clientId = "Your Client ID Here";
const clientSecret = "Your Client Secret Here";
const imageUrl = "https://path/to/image";
axios
.post("https://api.skybiometry.com/fc/faces/detect", {
api_key: clientId,
api_secret: clientSecret,
urls: imageUrl,
})
.then((response) => console.log(response.data))
.catch((error) => console.log(error));
Recognize Faces
The Recognize Faces endpoint compares an image against a previously stored gallery or against the set of detected faces from the Detect Faces API.
POST https://api.skybiometry.com/fc/faces/recognize
Example Code in JavaScript
const axios = require("axios");
const clientId = "Your Client ID Here";
const clientSecret = "Your Client Secret Here";
const imageUrl = "https://path/to/image";
const galleryName = "Your Gallery Name";
axios
.post("https://api.skybiometry.com/fc/faces/recognize", {
api_key: clientId,
api_secret: clientSecret,
urls: imageUrl,
namespace: galleryName,
})
.then((response) => console.log(response.data))
.catch((error) => console.log(error));
Create a Gallery
Create a Gallery endpoint creates a new gallery where you can store faces.
POST https://api.skybiometry.com/fc/galleries/create
Example Code in JavaScript
const axios = require("axios");
const clientId = "Your Client ID Here";
const clientSecret = "Your Client Secret Here";
const galleryName = "Your Gallery Name";
axios
.post("https://api.skybiometry.com/fc/galleries/create", {
api_key: clientId,
api_secret: clientSecret,
name_space: galleryName,
})
.then((response) => console.log(response.data))
.catch((error) => console.log(error));
Add Face to Gallery
Add a Face to Gallery endpoint adds a face to a previously created gallery.
POST https://api.skybiometry.com/fc/faces/recognize
Example Code in JavaScript
const axios = require("axios");
const clientId = "Your Client ID Here";
const clientSecret = "Your Client Secret Here";
const imageUrl = "https://path/to/image";
const galleryName = "Your Gallery Name";
const faceId = "Unique Identifier for the Face";
axios
.post("https://api.skybiometry.com/fc/faces/recognize", {
api_key: clientId,
api_secret: clientSecret,
urls: imageUrl,
name_space: galleryName,
uid: faceId,
})
.then((response) => console.log(response.data))
.catch((error) => console.log(error));
Conclusion
SkyBiometry provides a powerful API that can help you build intelligent applications that detect and recognize faces. This article explored some of the core API endpoints, and showed example code in JavaScript. For more information about this API, check out the SkyBiometry documentation.