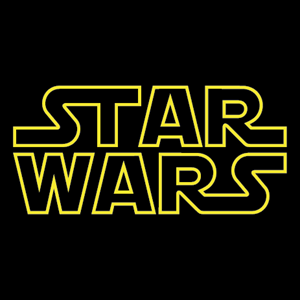
Star Wars API
Open DataStar Wars API with tons of information on the Star Wars universe. API lists you the planets, spaceships, vehicles, people, films and species. Form all Sever Star War films. Also has the "force awakens" data. You get hair color, skin color and eye color of the mentioned characters.
📚 Documentation & Examples
Everything you need to integrate with Star Wars API
🚀 Quick Start Examples
// Star Wars API API Example
const response = await fetch('https://swapi.dev/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Public API Docs: SWAPI
The Star Wars API (SWAPI) is a public API that provides data from the Star Wars universe, including information on characters, films, planets, and more.
Getting started
To start using the SWAPI, you'll need to make requests to its URL endpoints. The base URL for SWAPI is https://swapi.dev/api/
. From here, you can access all the available resources by adding the appropriate endpoint to the URL.
For example, to get information on the first Star Wars film, A New Hope, you would make a GET request to https://swapi.dev/api/films/1/
.
Example code in JavaScript
To make requests to the SWAPI in JavaScript, you can use tools like the fetch
API or libraries like axios
. Here are some example snippets of code that illustrate how to get data from the SWAPI:
Using the fetch
API
const url = "https://swapi.dev/api/films/1/";
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Using axios
const axios = require('axios');
const url = "https://swapi.dev/api/films/1/";
axios.get(url)
.then(response => console.log(response.data))
.catch(error => console.log(error));
Pagination
SWAPI uses pagination to limit the amount of data returned in a single request. You can specify the page number by adding a page
parameter to the URL.
For example, to get the second page of Star Wars characters, you would make a GET request to https://swapi.dev/api/people/?page=2
.
Here's some example code that shows how to get all data by paginating through the results:
const url = "https://swapi.dev/api/people/";
let allData = [];
function fetchData(url) {
axios.get(url)
.then(response => {
allData = [...allData, ...response.data.results];
if (response.data.next) {
fetchData(response.data.next);
} else {
console.log(allData);
}
})
.catch(error => console.log(error));
}
fetchData(url);
Conclusion
In this blog post, we've covered the basics of using the SWAPI, including how to make requests to its API endpoints in JavaScript, as well as how to handle pagination to get all available data.
With this knowledge, you can start exploring all the amazing data available in the Star Wars universe through the SWAPI.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes