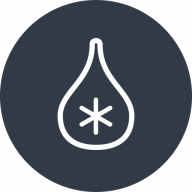
Storm Glass Marine Weather
WeatherDiscover the Weather with Stormglass API
If you're working on a project or app that requires weather data, Stormglass is a reliable and easy-to-use API that can help you fetch and analyze weather-related information.
Here are some examples of how to use the Stormglass API in JavaScript:
1. Installing the package
First, you need to install the Stormglass package using NPM:
npm install stormglass --save
2. Importing the package
To use the Stormglass API in your JavaScript file, you need to import the package like this:
const StormGlass = require('stormglass');
3. Setting up the API token
To access the Stormglass endpoints, you need to set up your API token as an environment variable. You can do that by creating a .env
file in your project's root directory and adding the following line:
STORMGLASS_API_KEY=<your API key here>
You can obtain your API key by creating an account on the Stormglass dashboard.
4. Fetching weather data
Once you've set up the package and your API token, you can use the forecastPoint()
method to fetch weather data for a specific location. The API requires you to provide a latitude, longitude, and time (in ISO 8601 format).
Here's an example of how to fetch weather data for San Francisco on January 1st, 2022:
const stormglass = new StormGlass({
key: process.env.STORMGLASS_API_KEY
});
const lat = 37.7749;
const lng = -122.4194;
const time = '2022-01-01T12:00:00Z';
stormglass.forecastPoint(lat, lng, time)
.then(response => {
// do something with the response
console.log(response);
})
.catch(err => {
// handle errors
console.error(err);
});
The response object will contain a variety of weather-related information, such as temperature, wind speed, wave height, and more.
5. Fetching weather data for multiple points
If you need to fetch weather data for multiple points, you can use the forecast()
method instead of forecastPoint()
. The forecast()
method requires you to provide an array of coordinates and times.
Here's an example of how to fetch weather data for San Francisco and Los Angeles on January 1st, 2022:
const stormglass = new StormGlass({
key: process.env.STORMGLASS_API_KEY
});
const points = [
{ lat: 37.7749, lng: -122.4194, time: '2022-01-01T12:00:00Z' },
{ lat: 34.0522, lng: -118.2437, time: '2022-01-01T15:00:00Z' }
];
stormglass.forecast(points)
.then(response => {
// do something with the response
console.log(response);
})
.catch(err => {
// handle errors
console.error(err);
});
The response object will contain an array of weather data objects, one for each point that you provided.
Conclusion
Overall, Stormglass is a straightforward and accessible API that can provide you with valuable weather-related information. By following the steps outlined above, you should be able to easily integrate the Stormglass API into your JavaScript projects and apps.