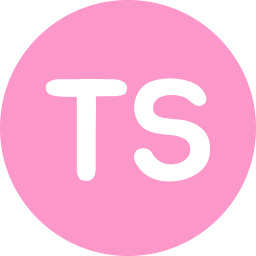
Taylor REST
Open DataExploring Taylor REST API
Taylor REST API provides a wide range of APIs that can be used to fetch data or perform actions. In this blog, we will explore some of the APIs and how to use them in JavaScript.
API Endpoints
The following are the API endpoints that are available with Taylor REST API:
- /about: Get information about Taylor REST API.
- /lorem: Generate random Lorem Ipsum text.
- /cat-fact: Get a random cat fact.
- /words: Get random words.
- /pokemon: Get a random Pokémon.
- /color: Get a random color.
- /advice: Get a random advice.
- /quote: Get a random quote.
- /joke: Get a random joke.
API Example Code in JavaScript
To make API requests in JavaScript, we can use the fetch()
method. The following code snippets show how to use some of the Taylor REST API endpoints:
// Get information about Taylor REST API
fetch('https://taylor.rest/about')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
// Generate random Lorem Ipsum text
fetch('https://taylor.rest/lorem')
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.error(error));
// Get a random cat fact
fetch('https://taylor.rest/cat-fact')
.then(response => response.json())
.then(data => console.log(data['fact']))
.catch(error => console.error(error));
// Get random words
fetch('https://taylor.rest/words')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
// Get a random Pokémon
fetch('https://taylor.rest/pokemon')
.then(response => response.json())
.then(data => console.log(data['name']))
.catch(error => console.error(error));
// Get a random color
fetch('https://taylor.rest/color')
.then(response => response.json())
.then(data => console.log(data['value']))
.catch(error => console.error(error));
// Get a random advice
fetch('https://taylor.rest/advice')
.then(response => response.json())
.then(data => console.log(data['advice']))
.catch(error => console.error(error));
// Get a random quote
fetch('https://taylor.rest/quote')
.then(response => response.json())
.then(data => console.log(data['quote']))
.catch(error => console.error(error));
// Get a random joke
fetch('https://taylor.rest/joke')
.then(response => response.json())
.then(data => console.log(data['joke']))
.catch(error => console.error(error));
Conclusion
In this blog, we explored some of the API endpoints available with Taylor REST API and saw how to make API requests in JavaScript. These APIs can be used to fetch data or perform actions, and can be integrated into various applications.