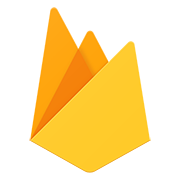
Text Recognition
Machine LearningUsing Firebase's ML Kit Recognize Text API with JavaScript
Firebase's ML Kit Recognize Text API allows you to extract text from images, whether it's handwritten or printed. In this blog post, we will explore how you can use this API with JavaScript.
Requirements
To use Firebase's ML Kit Recognize Text API, you will need to create a Firebase project and enable the ML Kit API. You can follow the instructions listed on the Getting Started guide to set this up.
Once you have set up your Firebase project and enabled the API, you will need to include the Firebase SDK in your JavaScript project by adding the following code to your HTML file:
<!-- Add Firebase libraries -->
<script src="https://www.gstatic.com/firebasejs/8.1.1/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/8.1.1/firebase-analytics.js"></script>
<script src="https://www.gstatic.com/firebasejs/8.1.1/firebase-messaging.js"></script>
<script src="https://www.gstatic.com/firebasejs/8.1.1/firebase-ml-vision.js"></script>
Recognizing Text
With the Firebase SDK included in your Javascript file, you can now use the firebase.ml().vision().cloudTextRecognizer()
method to recognize text in an image. Below is an example code snippet:
// Initialize Firebase
firebase.initializeApp(firebaseConfig);
// Get reference to image element
const img = document.getElementById("img");
const recognizeText = async () => {
// Create new ML Vision Cloud Text Recognizer instance
const textRecognizer = firebase
.ml()
.vision()
.cloudTextRecognizer();
// Pass image element as input
const result = await textRecognizer.processImage(img);
// Get text from recognized text object
const text = result.text;
// Log recognized text
console.log(text);
};
// Call recognizeText function
recognizeText();
The processImage()
method takes an image element as input and returns a promise that resolves to a cloudTextRecognition
object. You can then access the recognized text using the text
property.
Conclusion
In this blog post, we covered how to use Firebase's ML Kit Recognize Text API with JavaScript to recognize text in images. If you want to explore more of Firebase's ML Kit APIs, check out the official Firebase ML Kit documentation.