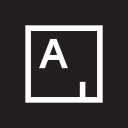
The Art World in Your App
Open DataExploring the Artsy API using JavaScript
Artsy is a platform for discovering and collecting art. Its API provides a wealth of information about artists, artworks, exhibitions, and more. In this blog post, we will explore the Artsy API using JavaScript and provide examples of how to access and use its resources.
Getting Started
First, we need to generate an Application on the Artsy API website (https://developers.artsy.net/docs/authentication).
Retrieving Data
We can retrieve data from the Artsy API by making GET requests to its endpoints. Here is an example of how to retrieve a list of artworks:
const apiKey = 'YOUR_API_KEY';
const endpoint = 'https://api.artsy.net/api/artworks';
fetch(endpoint, {
headers: {
'Accept': 'application/vnd.artsy-v2+json',
'X-Xapp-Token': apiKey,
}
})
.then(response => response.json())
.then(data => console.log(data));
In this code, we first define our API key and the endpoint that we want to access. Then, we use the fetch
function to make a GET request to the endpoint, specifying our API key in the X-Xapp-Token
header. When we get the response, we parse the JSON data and log it to the console.
Searching for Resources
Artsy API allows searching via several endpoints. Here is an example of searching for artists:
const apiKey = 'YOUR_API_KEY';
const endpoint = 'https://api.artsy.net/api/search?q=Andy Warhol&type=artist';
fetch(endpoint, {
headers: {
'Accept': 'application/vnd.artsy-v2+json',
'X-Xapp-Token': apiKey,
}
})
.then(response => response.json())
.then(data => console.log(data));
In this code, we make a GET request to the search endpoint, passing the search query q
and the resource type type
as parameters. The result of this request will be a list of artists that match the search query.
Conclusion
We have seen how to use JavaScript to access and search the Artsy API. The API offers a rich collection of data to work with, and the possibilities are endless. By using the examples in this post, you can start building your own projects using the Artsy API and exploring the world of art in new and exciting ways.