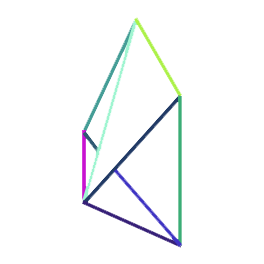
The Color API
Art & DesignWorking with The Color API
The Color API is a public API that allows developers to retrieve information about colors. The API is easy to use and provides plenty of examples to help you get started. In this tutorial, we will cover the basics of working with this API.
Getting Started
To get started using The Color API, you will need an API key. You can get one by signing up for a free account on their website. Once you have an API key, you can start making requests to the API.
Examples
Let's take a look at some example code in JavaScript that demonstrates how to use The Color API.
Get Color Information
This example shows how to retrieve information about a color using The Color API.
const colorCode = "FF0000"; // Red
fetch(`https://www.thecolorapi.com/id?hex=${colorCode}&format=json`)
.then(response => response.json())
.then(data => {
console.log(`Name: ${data.name.value}`);
console.log(`RGB: ${data.rgb.value}`);
console.log(`HSL: ${data.hsl.value}`);
});
Get Color Schemes
This example shows how to retrieve color schemes that include a specific color using The Color API.
const colorCode = "FF0000"; // Red
fetch(`https://www.thecolorapi.com/scheme?hex=${colorCode}&format=json`)
.then(response => response.json())
.then(data => {
for (let scheme of data.colors) {
console.log(`${scheme.name}: ${scheme.hex.value}`);
}
});
Get Random Colors
This example shows how to retrieve a random color using The Color API.
fetch(`https://www.thecolorapi.com/random?format=json`)
.then(response => response.json())
.then(data => {
console.log(`Name: ${data.name.value}`);
console.log(`RGB: ${data.rgb.value}`);
console.log(`HSL: ${data.hsl.value}`);
});
Conclusion
The Color API is an easy-to-use public API that provides plenty of information about colors. With the examples provided above, you should have a good understanding of how to retrieve color information, color schemes, and random colors from this API.