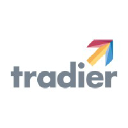
Tradier
FinanceThe US equity and options market data API offers comprehensive access to delayed, intraday, and historical market information, empowering developers and businesses to stay updated with real-time trading activities. This API from Tradier provides users with insights into the financial markets, enabling them to analyze trends, make informed investment decisions, and enhance their financial applications. With easy access to a wealth of data, users can explore historical price movements, track current market performance, and monitor option chain details, which can significantly elevate the analytical capabilities of trading platforms and financial software.
Utilizing the US equity/option market data API comes with several advantages. Users can benefit from seamless integration of market data into their applications, ensure reliability with access to historical and real-time information, and leverage customizable endpoints to cater to specific trading needs. Furthermore, the API is designed to support extensive data queries that help enhance trading strategies, while robust documentation ensures a smooth development process.
- Access to delayed, intraday, and historical market data
- Real-time updates for equities and options
- Comprehensive coverage of the US equity market
- Customizable endpoints for specific trading requirements
- Detailed documentation for easier integration and usage
Here’s a JavaScript example demonstrating how to call the API to fetch delayed market data:
const axios = require('axios');
const API_KEY = 'your_api_key_here';
const SYMBOL = 'AAPL'; // Stock symbol
async function getMarketData() {
try {
const response = await axios.get(`https://api.tradier.com/v1/markets/quotes`, {
headers: {
Authorization: `Bearer ${API_KEY}`,
Accept: 'application/json'
},
params: {
symbols: SYMBOL
}
});
console.log(response.data);
} catch (error) {
console.error('Error fetching market data:', error);
}
}
getMarketData();