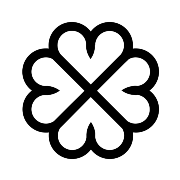
Trefle
EnvironmentIntroduction to Trefle.io API
Trefle.io API is a free and open-source plant database API. It has an extensive collection of plant information including common and scientific names, images, and other useful plant-related data. In this blog post, we will explore how to use the Trefle.io API to search and retrieve plant data using JavaScript.
How to access the Trefle.io API
To access the Trefle.io API, you need to sign up for an API key. The API key is used to authorize your requests to the Trefle.io API. You can sign up for an API key at https://trefle.io/.
Once you have the API key, you can start making requests to the Trefle.io API.
Searching for plants
To search for plants using the Trefle.io API, you can use the /plants/search
endpoint. This endpoint allows you to search for plants by their common or scientific names.
Here's an example code snippet in JavaScript that shows how to search for plants using the Trefle.io API:
const API_KEY = 'your-api-key';
const SEARCH_TERM = 'rose';
fetch(`https://trefle.io/api/v1/plants/search?token=${API_KEY}&q=${SEARCH_TERM}`)
.then(response => response.json())
.then(data => {
console.log(data);
});
In this code snippet, we first define our API key and search term. We then use the fetch
function to make a GET request to the /plants/search
endpoint with our API key and search term.
The response from the Trefle.io API is returned as a JSON object that contains an array of plants matching our search term.
Retrieving plant details
To retrieve details about a specific plant, you can use the /plants/:id
endpoint. This endpoint allows you to retrieve detailed information about a plant based on its Trefle.io ID.
Here's an example code snippet in JavaScript that shows how to retrieve plant details using the Trefle.io API:
const API_KEY = 'your-api-key';
const PLANT_ID = 12345;
fetch(`https://trefle.io/api/v1/plants/${PLANT_ID}?token=${API_KEY}`)
.then(response => response.json())
.then(data => {
console.log(data);
});
In this code snippet, we first define our API key and plant ID. We then use the fetch
function to make a GET request to the /plants/:id
endpoint with our API key and plant ID.
The response from the Trefle.io API is returned as a JSON object that contains detailed information about the specific plant we requested.
Conclusion
In this blog post, we explored how to use the Trefle.io API to search and retrieve plant data using JavaScript. The Trefle.io API is a powerful tool for anyone interested in plants and provides a wealth of information that can be easily accessed through JavaScript.