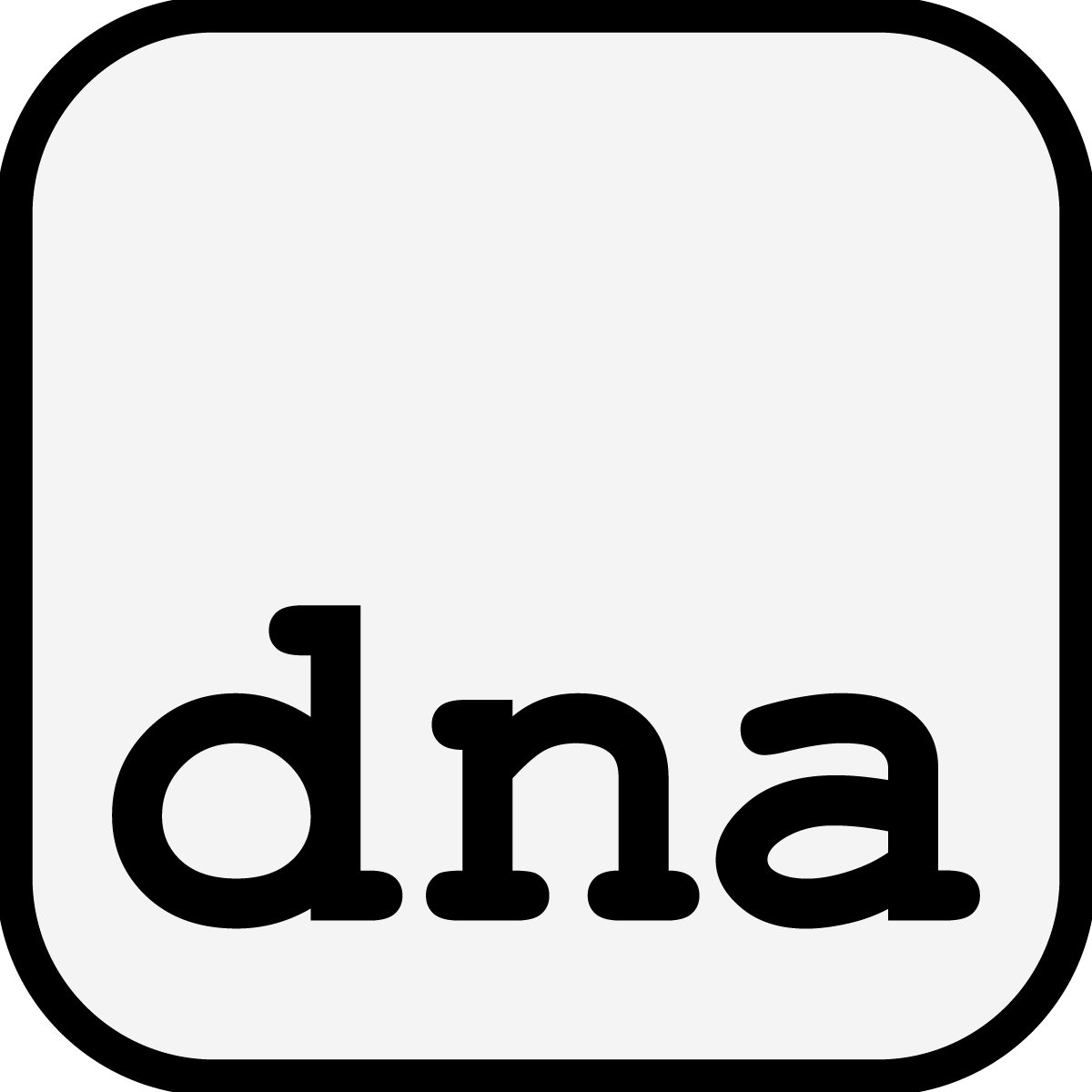
TypingDNA Authentication API
SecurityTypingDNA API Documentation
If you are building an application that needs to verify a user's identity based on their typing patterns, TypingDNA can provide you with the tools you need. With the TypingDNA API, you can easily integrate typing biometrics into your application.
Getting Started
To use the TypingDNA API, you will need to sign up for a developer account and get an API key. You can sign up on the TypingDNA website here. Once you have an API key, you can start using the TypingDNA API.
API Reference
Verify Typing Pattern
You can use the verify
method to verify a typing pattern against a previously recorded pattern.
const fetch = require('node-fetch');
const apiKey = 'YOUR_API_KEY';
const apiEndpoint = 'https://api.typingdna.com/verify';
const typingPattern = 'USER_TYPING_PATTERN';
const previousTypingPattern = 'PREVIOUS_TYPING_PATTERN';
fetch(apiEndpoint, {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded',
'Authorization': `Basic ${Buffer.from(apiKey).toString('base64')}`,
},
body: `tp=${typingPattern}&tp1=${previousTypingPattern}`,
})
.then(response => response.json())
.then(data => console.log(data));
Enroll Typing Pattern
You can use the save
method to save a user's typing pattern to the TypingDNA server.
const fetch = require('node-fetch');
const apiKey = 'YOUR_API_KEY';
const apiEndpoint = 'https://api.typingdna.com/save';
const typingPattern = 'USER_TYPING_PATTERN';
const userID = 'USER_ID';
fetch(apiEndpoint, {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded',
'Authorization': `Basic ${Buffer.from(apiKey).toString('base64')}`,
},
body: `tp=${typingPattern}&user=${userID}`,
})
.then(response => response.json())
.then(data => console.log(data));
Conclusion
The TypingDNA API provides a simple way to integrate typing biometrics into your application. With the ability to verify typing patterns, you can ensure that your users are who they say they are. By enrolling typing patterns, you can track individual users and detect changes in their typing patterns over time. Get started with the TypingDNA API today and add a new layer of security to your application.