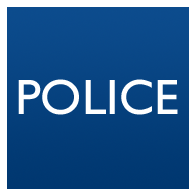
UK Police
SecurityExploring UK Police Data through APIs with JavaScript
APIs have made data access and analysis easier than ever before. One such fascinating API is the UK Police Data API which has a vast collection of publicly available data related to crime committed in the United Kingdom. This API can be accessed through the data.police.uk/docs website. In this blog post, we will learn how to make API calls and extract valuable information using JavaScript.
Getting Started
Before we start, let's take a look at the various datasets available through the API.
- Forces - Information about police forces and their senior officers
- Neighbourhoods - Information about all the neighbourhood policing areas for each police force
- Crimes at Street Level - Detailed crime information for specific streets and neighbourhoods
- Crimes at a Summary Level - Summarized crime information for specific areas like neighborhoods, constituencies, parliamentary constituencies, or the entire United Kingdom
To access any of these datasets, we will need to make an API request to the endpoints provided in the documentation.
Making API Requests using JavaScript
The easiest way to make an API request in JavaScript is to use the XMLHttpRequest object. Here's a sample code that shows how to make a GET request to the "All Forces" endpoint using this object.
const xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState === 4 && this.status === 200) {
const response = JSON.parse(this.responseText);
console.log(response);
}
};
xhttp.open("GET", "https://data.police.uk/api/forces", true);
xhttp.send();
In the above code, we have created an instance of the XMLHttpRequest object and set its properties and methods to make a GET request to the API. The readyState
property checks the state of the response, and the status
property checks the response status code. If the response is successful (status 200), the response is parsed as JSON and displayed in the console.
Extracting Data from the Response
Once we make a successful API request and get the response, we can extract the relevant data from it. Let's take the example of the "All Crimes" endpoint. The API documentation states that this endpoint returns information about all crimes within a given area for a specific month. Let's say we want to extract the crime category and street name for all the crimes in an area for a particular month.
const xhttp = new XMLHttpRequest();
const lat = "51.509865";
const lng = "-0.118092";
const date = "2020-12";
xhttp.onreadystatechange = function() {
if (this.readyState === 4 && this.status === 200) {
const response = JSON.parse(this.responseText);
response.forEach(crime => {
console.log(crime.category);
console.log(crime.location.street.name);
});
}
};
xhttp.open("GET", `https://data.police.uk/api/crimes-street/all-crime?lat=${lat}&lng=${lng}&date=${date}`, true);
xhttp.send();
In the above code, we are making a GET request to the "All Crimes" endpoint and passing the latitude, longitude, and date as query parameters. Once we get the response, we loop through each crime in the response and extract the crime category and street name.
Conclusion
In this blog post, we learned how to make API requests to the UK Police Data API using JavaScript and extract valuable information from the response. There are various datasets available through the API, and we can explore them further using similar techniques.
Did you find this post helpful? Have any questions or comments? Let us know in the comments section below!