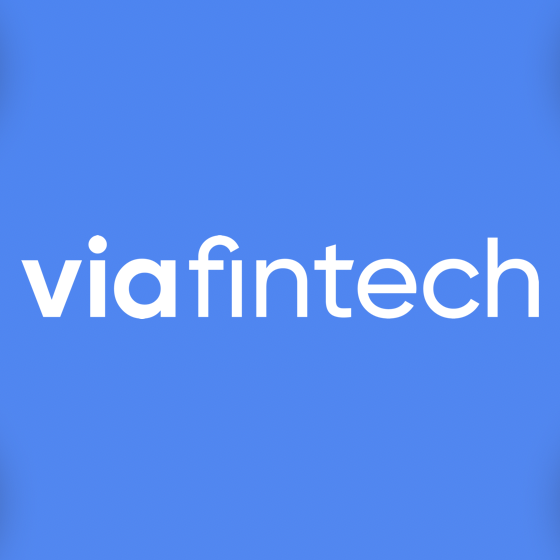
Viafintech
FinanceUsing the VIA Fintech API in JavaScript
If you are looking to integrate the VIA Fintech API into your JavaScript application, this guide is for you.
First, you'll need to sign up for an account and obtain your API keys. Once you have your API keys, you can start making requests to the VIA Fintech API.
Making Requests
You can use the built-in fetch
method to make requests to the VIA Fintech API.
const apiKey = 'your-api-key';
const endpoint = 'https://your-api-endpoint.com/api';
fetch(`${endpoint}/transactions`, {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`,
},
})
.then(response => response.json())
.then(data => console.log(data));
This example makes a GET
request to the transactions endpoint, passing in your API key as authorization. The response is then parsed as JSON and logged to the console.
Example Code
Here are some example uses of the VIA Fintech API in JavaScript.
Getting a list of transactions:
const apiKey = 'your-api-key';
const endpoint = 'https://your-api-endpoint.com/api';
fetch(`${endpoint}/transactions`, {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`,
},
})
.then(response => response.json())
.then(data => console.log(data));
Getting a specific transaction:
const apiKey = 'your-api-key';
const endpoint = 'https://your-api-endpoint.com/api';
const transactionId = 'transaction-id';
fetch(`${endpoint}/transactions/${transactionId}`, {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`,
},
})
.then(response => response.json())
.then(data => console.log(data));
Creating a new transaction:
const apiKey = 'your-api-key';
const endpoint = 'https://your-api-endpoint.com/api';
fetch(`${endpoint}/transactions`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`,
},
body: JSON.stringify({
amount: 100.00,
currency: 'USD',
recipient: 'john@example.com',
}),
})
.then(response => response.json())
.then(data => console.log(data));
Updating an existing transaction:
const apiKey = 'your-api-key';
const endpoint = 'https://your-api-endpoint.com/api';
const transactionId = 'transaction-id';
fetch(`${endpoint}/transactions/${transactionId}`, {
method: 'PATCH',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`,
},
body: JSON.stringify({
amount: 200.00,
currency: 'EUR',
}),
})
.then(response => response.json())
.then(data => console.log(data));
Deleting a transaction:
const apiKey = 'your-api-key';
const endpoint = 'https://your-api-endpoint.com/api';
const transactionId = 'transaction-id';
fetch(`${endpoint}/transactions/${transactionId}`, {
method: 'DELETE',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`,
},
})
.then(response => console.log(response.status === 204 ? 'Transaction deleted.' : 'Delete failed.' ));
Conclusion
The VIA Fintech API provides a powerful set of tools for managing transactions and other financial operations. By using the fetch
method in JavaScript, you can easily integrate the API into your applications.
Remember to always handle errors and check for valid responses when using any API.