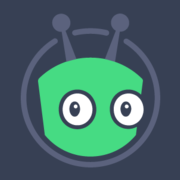
Vidyard
VideoExploring Vidyard Public APIs with JavaScript
Do you know that Vidyard has a comprehensive set of public APIs for developers to integrate with their platforms easily? These APIs are well-documented, secure, and easy to use, making it easy to interact with videos and related data programmatically.
In this article, we'll explore some of the essential Vidyard APIs and how to use them with JavaScript. Let's get started.
Getting Started with Vidyard API
Before we move on, ensure that you have an API key to access the Vidyard API. You can obtain your keys through the Vidyard Dashboard, under the Integrations tab. Once you have your key, you can now start making requests to the Vidyard API.
Retrieving Information with the Vidyard Player API
The Vidyard Player API enables you to embed the Vidyard player onto your website, access and control player-related data, and retrieve viewer data. Here's how to retrieve the video and player details of an embedded Vidyard player using the fetch
method:
fetch('https://api.vidyard.com/dashboard/v1/players/<player id>', {
method: 'GET',
headers: {
'Authorization': 'Bearer <API key>',
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data));
Uploading Videos with the Vidyard Upload API
The Vidyard Upload API allows you to upload videos programmatically. Here's how to upload a video using the API with the FormData
object:
const formData = new FormData();
formData.append('<file_key>', <File object>);
formData.append('payload', JSON.stringify({
title: '<video title>',
description: '<video description>',
tags: ['<tag 1>', '<tag 2>'],
player_id: '<player id>',
privacy: 'anybody',
notify: false,
cpu: 'none'
}));
fetch('https://api.vidyard.com/dashboard/v1/videos', {
method: 'POST',
headers: {
'Authorization': 'Bearer <API key>'
},
body: formData
})
.then(response => response.json())
.then(data => console.log(data));
Retrieving Analytics Data with the Vidyard Analytics API
The Vidyard Analytics API enables you to retrieve viewer data from your Vidyard videos in real-time. Here's how to retrieve viewer data using the API with the fetch
method:
fetch('https://api.vidyard.com/dashboard/v1/analytics/players/<player id>/videos/<video id>/events', {
method: 'GET',
headers: {
'Authorization': 'Bearer <API key>',
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data));
Conclusion
In conclusion, Vidyard's public APIs are easy to use and well-documented, making them ideal for developers that want to integrate video into their platforms seamlessly. With the examples we've covered here, you can now explore more Vidyard APIs and create powerful integrations.
👋 Happy coding!