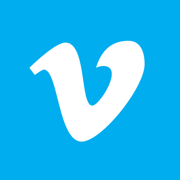
Vimeo
VideoVimeo Developer API. Vimeo's API supports flexible, high-quality video integration with your custom apps. Enjoy a full-featured upload API: privacy controls, flexible storage, and automatic transcoding to host videos in the highest quality. Optimize your experience with a fully customizable player (with SDKs), and third-party links for web, mobile, or TV. Manage content, track metadata, and optimize videos with the powerful features included in your Vimeo membership. Join the most passionate video community on the planet, and integrate features that connect millions of creators around the world.
📚 Documentation & Examples
Everything you need to integrate with Vimeo
🚀 Quick Start Examples
// Vimeo API Example
const response = await fetch('https://developer.vimeo.com/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring the Vimeo API with JavaScript
Vimeo is a popular video-sharing platform that hosts millions of videos across various categories. If you're a developer looking to build applications around video content, Vimeo offers a robust API that provides programmatic access to its features. In this article, we'll explore how to use the Vimeo API with JavaScript to perform actions like:
- Retrieving a list of videos
- Uploading videos
- Editing video metadata
- Embedding videos in your web app
Setting up your API access token
Before we can start interacting with the Vimeo API using JavaScript, we need to create an App on Vimeo Developer platform and generate an Access Token. You can generate an access token by creating a new app on Vimeo developer dashboard and follow the steps to get the access token.
Once you have generated the Access Token, you can include it in your JavaScript code to authenticate your API requests.
const ACCESS_TOKEN = "YOUR_ACCESS_TOKEN_HERE";
Retrieving a list of videos
To retrieve a list of videos from Vimeo, we can use the GET /me/videos
API endpoint. This endpoint returns a list of videos uploaded by the authenticated user.
const url = "https://api.vimeo.com/me/videos";
fetch(url, {
method: "GET",
headers: {
Authorization: `Bearer ${ACCESS_TOKEN}`,
},
})
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
In the above example, we're using the fetch
function to make a HTTP GET request to the https://api.vimeo.com/me/videos
endpoint with the Authorization header set to include the Access Token value. The response is parsed to JSON and output to the console.
Uploading videos
To upload a new video to Vimeo, we can use the POST /me/videos
API endpoint. This endpoint accepts a form data object containing the video file and other metadata like title, description, privacy setting, etc.
const url = "https://api.vimeo.com/me/videos";
const videoFile = document.getElementById("video-file").files[0];
const formData = new FormData();
formData.append("file", videoFile);
formData.append("name", "Sample video");
formData.append("description", "This is a sample video uploaded via Vimeo API");
formData.append("privacy.view", "nobody");
fetch(url, {
method: "POST",
headers: {
Authorization: `Bearer ${ACCESS_TOKEN}`,
},
body: formData,
})
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
In the above example, we're using the fetch
function to make a HTTP POST request to the https://api.vimeo.com/me/videos
endpoint with the Authorization header set to include the Access Token value and form data object containing video file and metadata fields.
Editing video metadata
To edit the metadata of an existing video on Vimeo, we can use the PATCH /videos/{video_id}
API endpoint. This endpoint accepts a JSON payload containing the updated values of the metadata fields.
const url = "https://api.vimeo.com/videos/12345";
const payload = JSON.stringify({
name: "New title",
description: "New description",
privacy: {
view: "anybody",
},
});
fetch(url, {
method: "PATCH",
headers: {
Authorization: `Bearer ${ACCESS_TOKEN}`,
"Content-Type": "application/json",
},
body: payload,
})
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
In the above example, we're using the fetch
function to make a HTTP PATCH request to the https://api.vimeo.com/videos/{video_id}
endpoint with the Authorization header set to include the Access Token value and JSON payload containing updated metadata fields.
Embedding videos in your web app
To embed a Vimeo video in your web app, you can use the Vimeo Player JavaScript API. This API provides a set of events and methods to control the playback of an embedded video.
<div id="vimeo-player"></div>
const player = new Vimeo.Player("vimeo-player", {
id: "12345",
});
player.on("play", function () {
console.log("Video is playing");
});
player.on("pause", function () {
console.log("Video is paused");
});
player.getDuration().then(function (duration) {
console.log("Video duration:", duration);
});
player.getThumbnailUrl().then(function (url) {
console.log("Video thumbnail URL:", url);
});
In the above example, we're creating a new Vimeo Player instance with the video ID and DOM element ID of where to embed the video. We're also listening to the play
and pause
events and retrieving the video duration and thumbnail URL using the getDuration
and getThumbnailUrl
methods respectively.
Conclusion
Using the Vimeo API with JavaScript, we can perform various operations on video content hosted on Vimeo. Whether you're building a video hosting platform or integrating video content within your application, the Vimeo API provides a powerful set of tools to accomplish your goals.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes