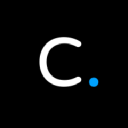
Virus Scan APIs
SecurityProtect Your Computer with Cloudmersive Virus API
With the increasing number of viruses and malware in the online world, it is essential to take the necessary steps to protect your computer or website. One way to do that is by using a virus detection and scanning tool. Cloudmersive Virus API is an efficient and easy-to-use tool that quickly detects and prevents malware attacks, making it one of the most popular virus detection tools available. In this blog post, we will explore the Cloudmersive Virus API and how to use it with JavaScript.
What is Cloudmersive Virus API?
Cloudmersive Virus API is a public API that performs virus scanning and detection tasks. It provides users with a simple and robust virus scanning tool that is capable of identifying a wide range of malware, including viruses, Trojans, and spyware. Cloudmersive Virus API's comprehensive library ensures it can identify a virus on Windows, Mac, and other operating systems.
How to Use Cloudmersive Virus API with JavaScript
A long list of API endpoints is available for Cloudmersive Virus API. However, in this blog post, we will discuss the most commonly used endpoints for virus scanning and detection.
Step 1: Sign up for Cloudmersive Virus API
To start using Cloudmersive Virus API, you will need to register for an API key. To do this, navigate to the Cloudmersive Virus API website, fill out the registration form, and submit it. You will receive an email from Cloudmersive containing your API key.
Step 2: Install the Cloudmersive API Client
There are several ways to install the Cloudmersive API client, depending on your project's setup. In this tutorial, we will use the npm package "@cloudmersive/virus-api-client."
npm install @cloudmersive/virus-api-client
Step 3: Scan a File
Now, let's write our first script to scan a file for viruses.
// Load the Cloudmersive Virus API client
const CloudmersiveVirusApiClient = require('@cloudmersive/virus-api-client');
// Replace YOUR_API_KEY with your actual API key
const defaultClient = CloudmersiveVirusApiClient.ApiClient.instance;
const Apikey = defaultClient.authentications['Apikey'];
Apikey.apiKey = 'YOUR_API_KEY';
const api = new CloudmersiveVirusApiClient.ScanApi();
// Replace 'filepath' with the path of the file you want to scan
const input = new CloudmersiveVirusApiClient.ScanFileRequest({ 'file': '/path/to/your/file' });
api.scanFile(input)
.then((data) => {
console.log('API called successfully. Response data: ' + data);
}, (error) => {
console.error(error);
});
The response data will contain the result of the virus scan, along with the list of viruses' names detected in the file, if any.
Step 4: Scan a URL
Cloudmersive Virus API also allows you to scan a URL for potential infections. Here's how you can do it.
// Load the Cloudmersive Virus API client
const CloudmersiveVirusApiClient = require('@cloudmersive/virus-api-client');
// Replace YOUR_API_KEY with your actual API key
const defaultClient = CloudmersiveVirusApiClient.ApiClient.instance;
const Apikey = defaultClient.authentications['Apikey'];
Apikey.apiKey = 'YOUR_API_KEY';
const api = new CloudmersiveVirusApiClient.ScanApi();
// Replace 'url' with a URL you want to scan
const input = new CloudmersiveVirusApiClient.ScanUrlRequest({ 'url': 'https://www.example.com' });
api.scanURL(input)
.then((data) => {
console.log('API called successfully. Response data: ' + data);
}, (error) => {
console.err(error);
});
Conclusion
In this blog post, we have learned about Cloudmersive Virus API, how to obtain an API key, and use it with JavaScript to scan a file or URL for malware. Remember, Cloudmersive Virus API offers a comprehensive virus detection solution; with its user-friendly interface, you can quickly integrate virus scanning capabilities into your application or website.