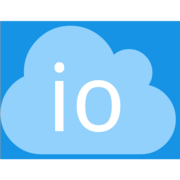
Weatherbit
WeatherExploring Weatherbit API with JavaScript
Weatherbit API is a powerful tool that allows developers to access real-time and historical weather data for any location on earth. In this blog, we will explore some of the Weatherbit API endpoints and their usage using JavaScript programming language.
Getting Started
To start using Weatherbit API, you need to sign up for an API key on the official website. Once you have your API key, you can start making requests to the API endpoints using JavaScript.
Current Weather
The Current Weather API endpoint returns the current weather conditions for a given location. Here is an example code in JavaScript that fetches the current weather information for New York City and logs it to the console:
const apiKey = 'YOUR_API_KEY';
const apiUrl = `https://api.weatherbit.io/v2.0/current?city=New%20York&country=USA&key=${apiKey}`;
async function getCurrentWeather() {
const response = await fetch(apiUrl);
const data = await response.json();
console.log(data);
}
getCurrentWeather();
This code uses the fetch
method to make a GET request to the Current Weather API endpoint. The async/await
syntax is used to handle the asynchronous nature of the API request.
Historical Weather
The Historical Weather API endpoint returns the historical weather data for a given location and time range. Here is an example code in JavaScript that fetches the historical weather information for New York City during the month of June 2021 and logs it to the console:
const apiKey = 'YOUR_API_KEY';
const apiUrl = `https://api.weatherbit.io/v2.0/history/monthly?city=New%20York&country=USA&start_date=2021-06-01&end_date=2021-06-30&key=${apiKey}`;
async function getHistoricalWeather() {
const response = await fetch(apiUrl);
const data = await response.json();
console.log(data);
}
getHistoricalWeather();
This code uses the fetch
method to make a GET request to the Historical Weather API endpoint. The start_date
and end_date
parameters are used to specify the time range for which historical data is required.
Forecast
The Forecast API endpoint returns the weather forecast for a given location and time range. Here is an example code in JavaScript that fetches the weather forecast for New York City for the next 7 days and logs it to the console:
const apiKey = 'YOUR_API_KEY';
const apiUrl = `https://api.weatherbit.io/v2.0/forecast/daily?city=New%20York&country=USA&days=7&key=${apiKey}`;
async function getForecast() {
const response = await fetch(apiUrl);
const data = await response.json();
console.log(data);
}
getForecast();
This code uses the fetch
method to make a GET request to the Forecast API endpoint. The days
parameter is used to specify the number of days for which the weather forecast is required.
Conclusion
Weatherbit API is a great tool for developers who want to access weather data for their projects. This blog explored some of the Weatherbit API endpoints and provided example code in JavaScript for each of them. With these examples, you should be able to start exploring more features of Weatherbit API and create your own weather-based applications.