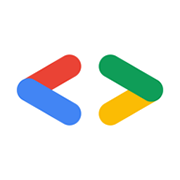
YouTube
VideoExploring Google's YouTube API
If you're building an application that involves video content, chances are you'll need to integrate with YouTube at some point. Fortunately, Google provides an extensive API that developers can use to interact with YouTube's features programmatically.
In this blog post, we'll take a look at some examples of how to use the YouTube API, focusing on JavaScript.
Getting Started
Before you can use the API, you'll need to obtain an API key from Google. This key will be used to authenticate your requests and track your usage. You can find instructions on how to obtain an API key in the official documentation.
Once you have your API key, you're ready to start making requests.
Searching for Videos
One of the most common use cases for the YouTube API is searching for videos based on certain criteria. Here's an example of how to perform a search using the API:
const API_KEY = 'YOUR_API_KEY';
const searchQuery = 'cat videos'; // replace with your own search term
const maxResults = 10; // maximum number of results to return
const endpoint = `https://www.googleapis.com/youtube/v3/search?part=snippet&maxResults=${maxResults}&q=${searchQuery}&key=${API_KEY}`;
fetch(endpoint)
.then(response => response.json())
.then(data => console.log(data));
This code fetches the first 10 videos that match the search query "cat videos" and logs the response data to the console. You can modify the searchQuery
and maxResults
variables to change the search term and the number of results to return.
Uploading Videos
Another powerful feature of the YouTube API is the ability to upload videos directly to YouTube from your application. Here's an example of how to upload a video using the API:
const API_KEY = 'YOUR_API_KEY';
const accessToken = 'ACCESS_TOKEN'; // replace with your own access token
const videoTitle = 'My Awesome Video'; // replace with your own video title
const videoDescription = 'This is a description of my awesome video.'; // replace with your own video description
const videoFile = new Blob(['VIDEO_DATA'], { type: 'video/mp4' }); // replace with your own video file data
const metadata = {
snippet: {
title: videoTitle,
description: videoDescription,
},
status: {
privacyStatus: 'unlisted', // video won't be public until you update the status
},
};
const endpoint = `https://www.googleapis.com/upload/youtube/v3/videos?part=snippet%2Cstatus&key=${API_KEY}`;
fetch(endpoint, {
method: 'POST',
headers: {
Authorization: `Bearer ${accessToken}`,
'Content-Type': 'application/json',
},
body: JSON.stringify(metadata),
})
.then(response => response.json())
.then(data => {
const { id } = data;
const uploadEndpoint = `https://www.googleapis.com/upload/youtube/v3/videos?part=snippet%2Cstatus%2CcontentDetails&id=${id}&key=${API_KEY}`;
return fetch(uploadEndpoint, {
method: 'POST',
headers: {
Authorization: `Bearer ${accessToken}`,
'Content-Type': 'video/mp4',
},
body: videoFile,
});
})
.then(response => console.log(response)); // logs the upload response
This code uploads a new video to YouTube with the title "My Awesome Video" and the description "This is a description of my awesome video." You'll need to replace the placeholders with your own values: the accessToken
variable should contain a valid access token that has been authorized to upload videos on behalf of the user, and the videoFile
variable should contain the actual video data in the form of a Blob
.
Note that the video will be "unlisted" by default, meaning it won't be publicly accessible until you change the video status to "public."
Conclusion
We've only scratched the surface of what's possible with the YouTube API in this blog post. If you're interested in learning more, be sure to check out the official documentation for a comprehensive guide to all the API features and examples in multiple programming languages.
With the power of the YouTube API at your fingertips, you can create dynamic, video-rich applications that engage and entertain users in entirely new ways.