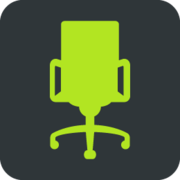
ZipRecruiter
JobsUsing the ZipRecruiter API in JavaScript
ZipRecruiter is a job board that lists job postings from various companies. They provide a public API that allows developers to get job listings, post jobs, and manage their job board account. In this blog post, we will explore how to use the ZipRecruiter API in JavaScript.
Getting Started
Before we can use the ZipRecruiter API, we need to sign up for a publisher account on their website. Once we have signed up, we will be given an API key that we can use to authenticate our requests.
Authentication
To authenticate our requests, we need to include our API key in the Authorization
header. Here's an example of how to do this in JavaScript:
const apiKey = '<your api key>';
const headers = { 'Authorization': `Bearer ${apiKey}` };
Getting Job Listings
To get job listings from the ZipRecruiter API, we can make a GET request to the https://api.ziprecruiter.com/jobs
endpoint. Here's an example of how to do this in JavaScript:
const searchParams = new URLSearchParams({
location: 'san francisco,ca',
distance: 10,
job_title: 'javascript developer'
});
const url = `https://api.ziprecruiter.com/jobs?${searchParams}`;
fetch(url, { headers })
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are searching for jobs in San Francisco, CA that have "javascript developer" in their job title and are within 10 miles of the location.
Posting Jobs
To post a job to the ZipRecruiter API, we can make a POST request to the https://api.ziprecruiter.com/job/create
endpoint. Here's an example of how to do this in JavaScript:
const job = {
title: 'Javascript Developer',
description: 'We are looking for a talented Javascript developer to join our team...',
company_name: 'Acme Inc.',
location: 'San Francisco, CA',
salary: '$100,000 - $120,000',
job_type: 'Full-Time'
};
fetch('https://api.ziprecruiter.com/job/create', {
method: 'POST',
headers,
body: JSON.stringify(job)
}).then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are posting a job with a title, description, company name, location, salary, and job type.
Conclusion
In this blog post, we explored how to use the ZipRecruiter API in JavaScript. We looked at how to authenticate our requests, get job listings, and post jobs. With these examples, you should be able to build your own job board or integrate ZipRecruiter into your existing application.